c++循环分支结构例题
时间: 2024-10-31 15:04:23 浏览: 21
在C++中,循环和分支结构用于控制程序的流程,常见的例子包括`for`、`while`、`do-while`循环以及`if`、`else`、`switch`等条件判断。下面是一个简单的示例,展示如何结合它们来遍历数组并根据元素值执行不同的操作:
```cpp
#include <iostream>
using namespace std;
int main() {
int array[] = {10, 20, 30, 40, 50};
int size = sizeof(array) / sizeof(array[0]);
for (int i = 0; i < size; ++i) {
if (array[i] % 2 == 0) { // 判断是否为偶数
cout << "Element " << i+1 << " is even: " << array[i] << endl;
} else { // 如果不是偶数
if (array[i] > 30) { // 再判断是否大于30
cout << "Element " << i+1 << " is odd and greater than 30: " << array[i] << endl;
} else {
cout << "Element " << i+1 << " is odd but less than or equal to 30: " << array[i] << endl;
}
}
}
return 0;
}
```
在这个例子中,我们首先用`for`循环遍历数组。然后,对于每个元素,如果它是偶数,就打印一条消息;如果不是,再通过`if-else`嵌套判断它是否大于30,从而执行相应的输出。
阅读全文
相关推荐



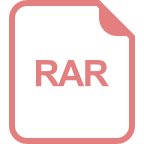



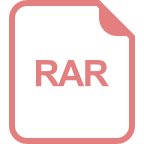


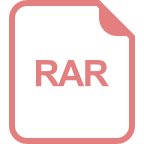








