用c++完成电瓶车租赁管理系统,包含增删改查,用到文件读写
时间: 2024-09-17 16:03:55 浏览: 54
在C++中创建一个简单的电动车租赁管理系统,可以利用文件I/O功能来存储数据。我们将创建一个类`ElectricBike`表示电动车,以及几个成员函数来处理租借(Rent)、归还(Return)、添加(Add)和删除(Delete)操作。这里是一个基本的框架:
```cpp
#include <iostream>
#include <fstream>
#include <string>
class ElectricBike {
private:
int bikeId;
std::string bikeName;
bool isRented;
public:
// 构造函数
ElectricBike(int id, const std::string& name) : bikeId(id), bikeName(name), isRented(false) {}
// 添加自行车到文件
static void addToFile(const ElectricBike& bike, const std::string& filename) {
std::ofstream file(filename, std::ios_base::app);
if (file.is_open()) {
file << bike.bikeId << "," << bike.bikeName << "," << bike.isRented << "\n";
file.close();
}
}
// 从文件中读取并返回所有自行车
static std::vector<ElectricBike> readFromFile(const std::string& filename) {
std::ifstream file(filename);
std::vector<ElectricBike> bikes;
if (file.is_open()) {
std::string line;
while (std::getline(file, line)) {
std::istringstream iss(line);
int id, rented;
std::string name;
iss >> id >> name >> rented;
bikes.push_back(ElectricBike(id, name, rented));
}
file.close();
}
return bikes;
}
// 执行操作(这里简化为打印信息)
void rent() { isRented = true; std::cout << "Bike " << bikeId << " has been rented.\n"; }
void returnBike() { isRented = false; std::cout << "Bike " << bikeId << " returned.\n"; }
};
int main() {
// 示例操作
ElectricBike bike1(1, "Eco-Electric");
ElectricBike::addToFile(bike1, "bikes.txt");
// 从文件读取并显示所有车辆状态
std::vector<ElectricBike> allBikes = ElectricBike::readFromFile("bikes.txt");
for (const auto& bike : allBikes) {
if (bike.isRented)
std::cout << "Bike " << bike.bikeId << " is currently rented.\n";
else
std::cout << "Bike " << bike.bikeId << " is available.\n";
}
return 0;
}
```
阅读全文
相关推荐
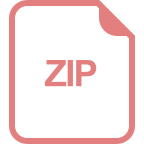
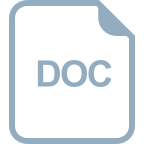
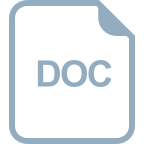
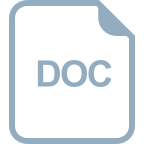
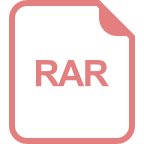
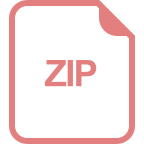
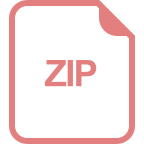
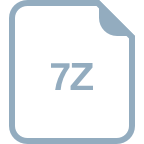
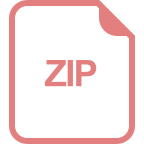
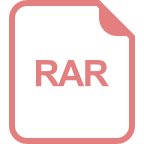
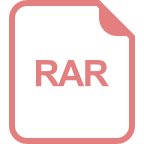
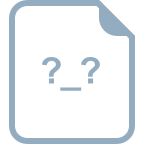
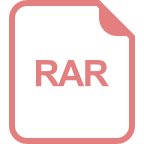
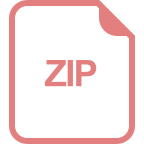
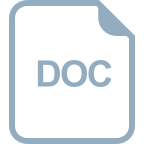