用python对带日期的时间进行加减
时间: 2024-05-16 16:19:07 浏览: 123
可以使用Python中的datetime模块来对带日期的时间进行加减操作。下面是一个示例:
```python
from datetime import datetime, timedelta
# 当前时间
current_time = datetime.now()
print("当前时间:", current_time)
# 加上一天
next_day = current_time + timedelta(days=1)
print("加上一天:", next_day)
# 减去一周
last_week = current_time - timedelta(weeks=1)
print("减去一周:", last_week)
# 加上两个小时
two_hours_later = current_time + timedelta(hours=2)
print("加上两个小时:", two_hours_later)
```
这个示例中,首先使用`datetime.now()`获取当前时间,然后使用`timedelta`来表示时间间隔。通过加减`timedelta`对象,可以对当前时间进行加减操作。在示例中,我们分别加了一天、减了一周、加了两个小时。最后打印出了计算后的时间。
请注意,这里的日期时间对象是基于系统当前时间的,如果你需要操作其他指定的日期时间,可以使用`datetime`的构造函数来创建特定的日期时间对象。
相关问题
python怎么进行日期的加减
在Python中,你可以使用内置的`datetime`模块来进行日期的加减操作。这个模块提供了一个名为`timedelta`的对象,可以方便地执行日期和时间的增量或减量。
以下是基本的日期加减示例:
```python
from datetime import datetime, timedelta
# 当前日期
current_date = datetime.now()
# 加上一定的时间
date_plus_days = current_date + timedelta(days=5) # 加五天
date_plus_hours = current_date + timedelta(hours=12) # 加十二小时
# 减去一定的时间
date_minus_days = current_date - timedelta(days=7) # 减七天
date_minus_hours = current_date - timedelta(hours=4) # 减四小时
print(date_plus_days, date_minus_hours)
```
在这个例子中,`timedelta`构造函数接受各种参数,如'days', 'hours', 'minutes', 'seconds', 'microseconds'等,用于指定时间间隔。
如果你想根据特定的日期和时间进行计算,可以直接传递两个`datetime`对象,它们之间的差异会被自动计算出来:
```python
start_date = datetime(2023, 1, 1)
end_date = datetime(2023, 1, 10)
delta = end_date - start_date
print(delta.days) # 输出9,即相隔9天
```
python日期加减
在 Python 中,可以使用 datetime 模块来进行日期的加减操作。下面是一些常见的日期加减操作示例:
1. 加减天数:
```python
from datetime import datetime, timedelta
# 当前日期
current_date = datetime.now()
# 加一天
next_day = current_date + timedelta(days=1)
# 减一天
previous_day = current_date - timedelta(days=1)
```
2. 加减小时、分钟、秒等:
```python
from datetime import datetime, timedelta
# 当前时间
current_time = datetime.now()
# 加一小时
next_hour = current_time + timedelta(hours=1)
# 减一分钟
previous_minute = current_time - timedelta(minutes=1)
# 加十秒
next_ten_seconds = current_time + timedelta(seconds=10)
```
3. 加减月份:
```python
from datetime import datetime
from dateutil.relativedelta import relativedelta
# 当前日期
current_date = datetime.now()
# 加一个月
next_month = current_date + relativedelta(months=+1)
# 减两个月
previous_two_months = current_date + relativedelta(months=-2)
```
请注意,以上示例中的日期加减操作都是基于当前日期或时间进行的。您可以根据具体需求,使用 timedelta 或 relativedelta 对象来进行日期加减操作。
希望这些示例能对您有所帮助!如果您还有其他问题,请随时提问。
阅读全文
相关推荐
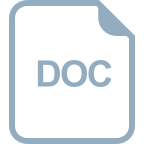
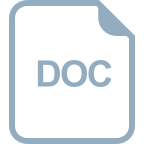
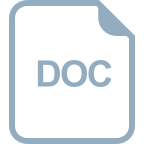
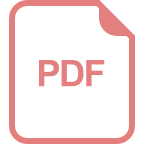







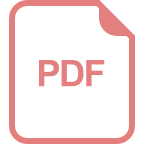
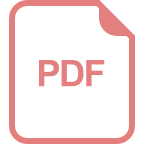
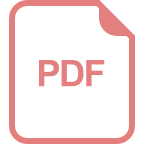
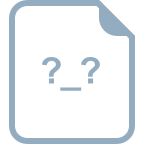

