Let 𝑃 be a set of 𝑛 points on the 𝑥-axis and each of the points is colored with one of the colors 1,2, . . . , 𝑘. For each color 𝑖 of the 𝑘 colors, there is at least one point in 𝑃 which is colored with 𝑖. For a set 𝑃 ′ of consecutive points from 𝑃, if both 𝑃 ′ and 𝑃 ∖ 𝑃 ′ contain at least one point of each color, then we say that 𝑃 ′ makes a double rainbow. See the below figure as an example. The set 𝑃 consists of ten points and each of the points is colored by one of the colors 1, 2, 3, and 4. The set 𝑃 ′ of the five consecutive points contained in the rectangle makes a double rainbow. Given a set 𝑃 of points and the number 𝑘 of colors as input, write a program that computes and prints out the minimum size of 𝑃 ′ that makes a double rainbow. Input Your program is to read from standard input. The input starts with a line containing two integers 𝑛 and 𝑘 (1 ≤ 𝑘 ≤ 𝑛 ≤ 10,000), where 𝑛 is the number of the points in 𝑃 and 𝑘 is the number of the colors. Each of the following 𝑛 lines consists of an integer from 1 to 𝑘, inclusively, and the 𝑖-th line corresponds to the color of the 𝑖-th point of 𝑃 from the left. Output Your program is to write to standard output. Print exactly one line. The line should contain the minimum size of 𝑃 ′ that makes a double rainbow. If there is no such 𝑃 ′ , print 0. The following shows sample input and output for two test cases.
时间: 2024-02-14 13:33:24 浏览: 48
Sure! Here's an example C++ code that solves the problem:
```cpp
#include <iostream>
#include <vector>
#include <unordered_set>
int findMinimumSizeOfDoubleRainbow(const std::vector<int>& colors, int k) {
int n = colors.size();
std::vector<int> colorCount(k + 1, 0);
std::unordered_set<int> leftColors;
int minSize = n + 1;
// Iterate over all possible sizes of P' from 1 to n
for (int size = 1; size <= n; size++) {
// Reset color counts and left colors for each size
std::fill(colorCount.begin(), colorCount.end(), 0);
leftColors.clear();
// Count colors in the first size elements
for (int i = 0; i < size; i++) {
colorCount[colors[i]]++;
leftColors.insert(colors[i]);
}
bool foundDoubleRainbow = true;
// Check if there exists a valid P' of size 'size'
for (int i = 0; i < n - size + 1; i++) {
// Check if all colors are present in both P' and P \ P'
if (leftColors.size() == k && colorCount == std::vector<int>(k + 1, 1)) {
minSize = std::min(minSize, size);
foundDoubleRainbow = true;
break;
}
// Update color counts and left colors
colorCount[colors[i]]--;
if (colorCount[colors[i]] == 0) {
leftColors.erase(colors[i]);
}
colorCount[colors[i + size]]++;
leftColors.insert(colors[i + size]);
}
if (!foundDoubleRainbow) {
break;
}
}
return (minSize == n + 1) ? 0 : minSize;
}
int main() {
int n, k;
std::cin >> n >> k;
std::vector<int> colors(n);
for (int i = 0; i < n; i++) {
std::cin >> colors[i];
}
int minSize = findMinimumSizeOfDoubleRainbow(colors, k);
std::cout << minSize << std::endl;
return 0;
}
```
You can compile and run this code to solve the problem. It reads the input from standard input and prints the minimum size of 𝑃 ′ that makes a double rainbow to standard output.
Note: This code assumes that the input is valid and follows the given constraints. You may need to add additional input validation if necessary.
相关推荐
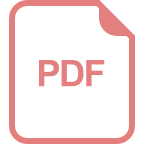
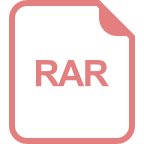













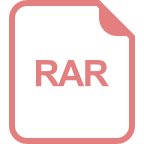
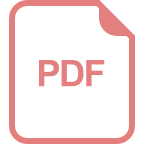