画决策树matlab代码
时间: 2023-09-13 09:06:11 浏览: 183
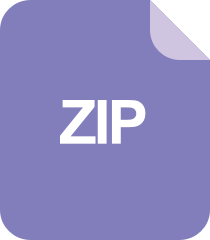
基于决策树DT的数据回归预测,多变量输入模型,matlab代码 评价指标包括:R2、MAE、MSE、RMSE和MAPE等,代

当然可以为您提供一个简单的决策树的 MATLAB 代码示例。以下是一个使用 MATLAB 的 fitctree 函数来创建和可视化决策树的示例代码:
```matlab
% 输入样本特征和标签
X = [1 0; 1 1; 0 1; 0 0]; % 样本特征
Y = [1; 1; 0; 0]; % 样本标签
% 创建和训练决策树模型
tree = fitctree(X, Y);
% 可视化决策树
view(tree, 'Mode', 'graph');
```
在这个例子中,我们的样本特征 `X` 是一个 4x2 的矩阵,其中每一行代表一个样本的特征。我们的样本标签 `Y` 是一个 4x1 的向量,其中每个元素对应于相应样本的标签。
使用 `fitctree` 函数可以创建和训练决策树模型。然后,使用 `view` 函数将决策树可视化为图形。
请注意,这只是一个简单的示例,真实场景中可能需要更多的数据预处理、参数调整等操作。此外,也可以使用其他的决策树算法或者库来创建决策树模型。
希望这个示例能对您有所帮助!如有任何问题,请随时提问。
阅读全文
相关推荐
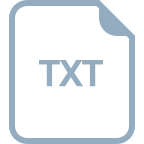
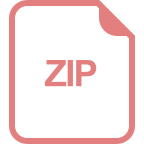
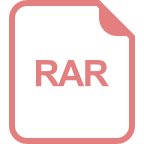
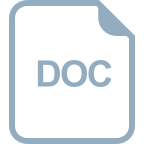


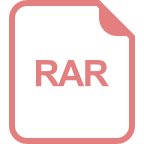
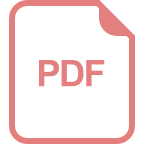
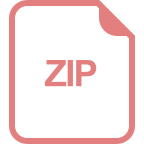
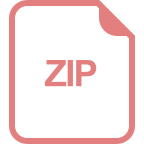
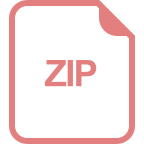
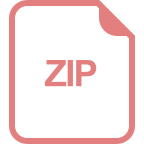
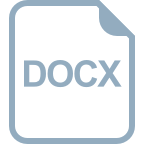
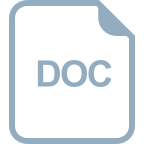
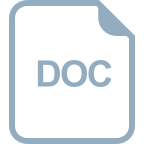
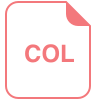
