如何在编程语言中使用正规式进行模式匹配?
时间: 2024-10-02 22:03:21 浏览: 42
在编程语言中使用正则表达式进行模式匹配是一种强大的文本处理技术。正则表达式(Regular Expression,简称regex)是一种描述字符序列的模式,可以用来匹配、查找、替换文本等操作。以下是一些常见的步骤和示例:
1. **导入正则表达式库**:不同的编程语言有不同的正则表达式库或模块。例如,在Python中,可以使用内置的`re`模块;在JavaScript中,可以使用全局对象`RegExp`。
```python
import re
```
2. **编写正则表达式**:根据需求编写适当的正则表达式。例如,匹配一个电子邮件地址的基本正则表达式可以是:
```regex
^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$
```
3. **使用正则表达式函数**:大多数编程语言提供了一组用于操作正则表达式的函数。常见的有匹配、查找、替换等。
- **匹配**:检查字符串是否与正则表达式匹配。
```python
if re.match(r'^\d+$', '12345'):
print("The string contains only digits.")
```
- **查找**:查找字符串中所有匹配的子串。
```python
matches = re.findall(r'\d+', 'There are 123 apples and 456 oranges.')
print(matches) # Output: ['123', '456']
```
- **替换**:替换字符串中匹配的子串。
```python
result = re.sub(r'\d+', '#', 'There are 123 apples and 456 oranges.')
print(result) # Output: There are # apples and # oranges.
```
4. **捕获组和反向引用**:使用圆括号`()`可以创建捕获组,用于提取匹配的子串。反向引用可以用来重复匹配之前捕获的内容。
```python
text = "Contact 123-456-7890 or email@example.com"
match = re.search(r'(\d{3})-(\d{3})-(\d{4})', text)
if match:
print(f"Phone number: {match.group(1)}-{match.group(2)}-{match.group(3)})
```
阅读全文
相关推荐



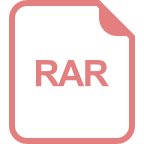
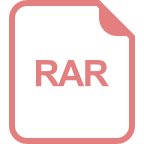




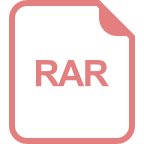








