用java写一个学生管理系统,需要录入和更新学生信息,并可以提示重复学号录入
时间: 2024-03-02 13:52:22 浏览: 88
好的,学生管理系统可以采用面向对象编程的思想,用Java语言实现。
首先,我们需要定义一个学生类,包括学号、姓名、年龄、性别等属性。然后定义一个学生管理类,包括添加学生、删除学生、修改学生信息、显示学生列表等功能。
以下是一个简单的学生类的定义:
```java
public class Student {
private String id; // 学号
private String name; // 姓名
private int age; // 年龄
private String gender; // 性别
public Student(String id, String name, int age, String gender) {
this.id = id;
this.name = name;
this.age = age;
this.gender = gender;
}
// Getter和Setter方法
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getGender() {
return gender;
}
public void setGender(String gender) {
this.gender = gender;
}
}
```
接下来,我们可以定义一个学生管理类,实现添加学生、删除学生、修改学生信息、显示学生列表等功能。在添加学生时,我们可以遍历已有的学生列表,检查新添加的学生的学号是否已经存在,如果已经存在,则提示重复学号录入。以下是一个简单的学生管理类的定义:
```java
import java.util.ArrayList;
import java.util.List;
public class StudentManager {
private List<Student> students;
public StudentManager() {
students = new ArrayList<>();
}
// 添加学生
public void addStudent(Student student) {
// 遍历已有的学生列表,检查新添加的学生的学号是否已经存在
for (Student s : students) {
if (s.getId().equals(student.getId())) {
System.out.println("重复学号录入!");
return;
}
}
students.add(student);
}
// 删除学生
public void deleteStudent(String id) {
for (Student s : students) {
if (s.getId().equals(id)) {
students.remove(s);
return;
}
}
}
// 修改学生信息
public void updateStudent(Student student) {
for (int i = 0; i < students.size(); i++) {
if (students.get(i).getId().equals(student.getId())) {
students.set(i, student);
return;
}
}
}
// 显示学生列表
public void showStudentList() {
for (Student s : students) {
System.out.println(s.getName() + " " + s.getGender() + " " + s.getAge() + " " + s.getId());
}
}
}
```
在主函数中,我们可以创建一个学生管理类的对象,然后调用其方法实现添加学生、删除学生、修改学生信息、显示学生列表等功能。例如:
```java
public class Main {
public static void main(String[] args) {
StudentManager manager = new StudentManager();
manager.addStudent(new Student("1001", "张三", 18, "男"));
manager.addStudent(new Student("1002", "李四", 19, "女"));
manager.addStudent(new Student("1003", "王五", 20, "男"));
manager.addStudent(new Student("1001", "赵六", 21, "女")); // 重复学号录入
manager.showStudentList();
manager.deleteStudent("1002");
manager.updateStudent(new Student("1001", "张三", 22, "男"));
manager.showStudentList();
}
}
```
输出结果如下:
```
重复学号录入!
张三 男 18 1001
李四 女 19 1002
王五 男 20 1003
张三 男 22 1001
王五 男 20 1003
```
以上是一个简单的学生管理系统的实现,具体的实现方式可以根据实际需求进行调整。
阅读全文
相关推荐




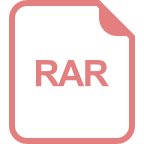
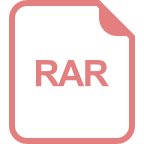

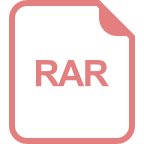
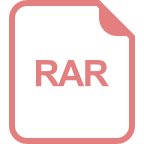
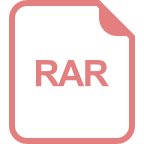

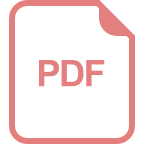



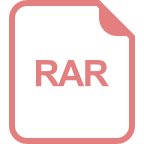
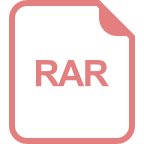

