vue3.0中使用echarts中的legend事件被外层的div事件遮挡,怎么解决
时间: 2024-02-21 08:58:08 浏览: 24
在 Vue3.0 中使用 ECharts,解决图例(legend)事件被外层 div 事件遮挡的问题,可以通过以下步骤实现:
1. 在 Vue3.0 中使用 ECharts,需要先安装 `echarts` 和 `vue-echarts` 两个依赖:
```bash
npm install echarts vue-echarts
```
2. 在 Vue3.0 中使用 ECharts,需要在组件中注册 ECharts 组件,并将图表配置项传递给 ECharts 组件:
```vue
<template>
<div class="chart-container">
<vue-echarts :options="chartOptions" @legend-selectchanged="handleLegendSelect"></vue-echarts>
</div>
</template>
<script>
import * as echarts from 'echarts';
import { use } from 'echarts/core';
import { CanvasRenderer } from 'echarts/renderers';
import { LineChart } from 'echarts/charts';
import { GridComponent, TooltipComponent, LegendComponent } from 'echarts/components';
import VueECharts from 'vue-echarts';
// 注册必要的组件
use([CanvasRenderer, LineChart, GridComponent, TooltipComponent, LegendComponent]);
export default {
components: {
VueECharts,
},
data() {
return {
// ECharts 图表配置项
chartOptions: {
// ...
},
};
},
methods: {
// 监听图例的选择事件
handleLegendSelect(params) {
console.log(params);
},
},
};
</script>
```
3. 在组件中,为外层 div 元素添加一个事件监听器,并通过判断事件的 target 是否为图例元素来决定是否处理该事件:
```vue
<template>
<div class="chart-container" @click="handleContainerClick">
<vue-echarts :options="chartOptions" @legend-selectchanged="handleLegendSelect"></vue-echarts>
</div>
</template>
<script>
import * as echarts from 'echarts';
import { use } from 'echarts/core';
import { CanvasRenderer } from 'echarts/renderers';
import { LineChart } from 'echarts/charts';
import { GridComponent, TooltipComponent, LegendComponent } from 'echarts/components';
import VueECharts from 'vue-echarts';
// 注册必要的组件
use([CanvasRenderer, LineChart, GridComponent, TooltipComponent, LegendComponent]);
export default {
components: {
VueECharts,
},
data() {
return {
// ECharts 图表配置项
chartOptions: {
// ...
},
};
},
methods: {
// 监听图例的选择事件
handleLegendSelect(params) {
console.log(params);
},
// 监听外层 div 的点击事件
handleContainerClick(event) {
if (event.target.tagName === 'SPAN' && event.target.className.indexOf('ec-legend-item') >= 0) {
// 如果点击的是图例元素,则处理该事件
event.stopPropagation();
this.$refs.chart.dispatchAction({
type: 'legendToggleSelect',
name: event.target.innerText,
});
}
},
},
};
</script>
```
在上述代码中,我们为外层 div 元素添加了一个 `@click` 事件监听器,并在事件处理函数中判断事件的 target 是否为图例元素。如果是,则处理该事件,并通过 `$refs.chart` 获取到 ECharts 实例,调用 `dispatchAction` 方法来触发图例的展示或隐藏。同时,我们在处理事件时调用了 `event.stopPropagation` 方法来阻止事件冒泡到外层 div,从而避免了被遮挡的问题。
相关推荐
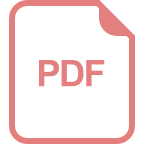













