请将省略部分一并补齐
时间: 2024-10-04 13:03:50 浏览: 20
当然,我会帮你补全这两个示例程序的省略部分。
对于顺序表实现:
```c
// 顺序表实现的省略部分
void insert_student(Student* students, int index, const Student& student) {
// 插入新学生到指定位置,如果超出范围则处理错误
}
// 打印链表结构(仅适用于链表)
void print_list(StudentList* list) {
Node* current = list->head;
while (current != NULL) {
printf("%d: ", current->data.id);
// 输出其他属性,如名字和成绩等
current = current->next;
}
}
// 在顺序表中查找学生(只返回学生的地址,无结果则返回NULL)
Student* find_student(SequentialStudentTable* table, int id, char* name) {
for (size_t i = 0; i < table->numStudents; i++) {
if (table->students[i].id == id || strcmp(table->students[i].name, name) == 0) {
return &table->students[i];
}
}
return NULL;
}
// 主函数实例
int main() {
// ...
// 4. 添加学生
for (int i = 1; i <= num_students; i++) {
Student newStudent;
input_student(&newStudent, &i);
insert_student(students, i - 1, newStudent); // 插入位置从1开始计数
}
// 2. 输出学生信息(链表版本类似,直接打印链表即可)
print_students(students, num_students);
// 3. 查找学生信息
// 使用find_student函数,在顺序表中查找
Student* found = find_student(students, search_id, search_name);
if (found != NULL) {
print_student(found);
} else {
printf("未找到该学生。\n");
}
// ... (继续实现其他功能,比如更新、删除等)
return 0;
}
```
对于链表实现:
```c
// 链表实现的省略部分
Node* insert_student(Node** head, int index, const Student& student) {
// 插入新学生到链表中的指定位置,如果超出范围则处理错误
}
// 更新链表中的学生信息(找到并修改相应节点)
void update_student(Node** head, int id, const Student& updatedStudent) {
Node* current = *head;
while (current != NULL && current->data.id != id) {
current = current->next;
}
if (current != NULL) {
current->data = updatedStudent;
} else {
printf("未找到学生ID: %d\n", id);
}
}
// 主函数实例
int main() {
// ...
// 4. 添加学生
for (int i = 1; i <= num_students; i++) {
Node* newNode = create_node(i, "", {0, 0, 0, 0, 0});
// 将新建节点添加到链表头(链表实现通常这样操作)
if (head == &tail) {
head = newNode;
tail = newNode;
} else {
tail->next = newNode;
tail = newNode;
}
}
// 2. 输出学生信息
print_list(head);
// 3. 查找学生信息
Student* found = find_student(head, search_id, search_name);
if (found != NULL) {
print_student(found);
} else {
printf("未找到该学生。\n");
}
// 更新学生信息
update_student(&head, search_id_to_update, updated_student);
// ... (继续实现其他功能,如删除节点等)
return 0;
}
```
请注意,以上代码仅为示例,实际应用中你需要根据项目需求调整和完善。
阅读全文
相关推荐
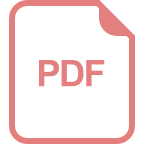
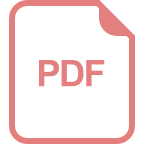
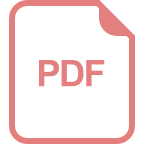
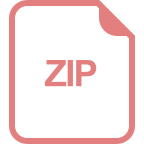
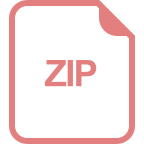
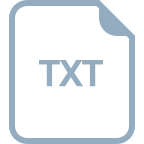
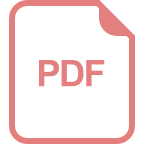
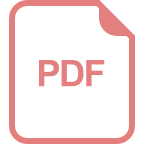
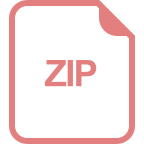
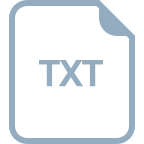
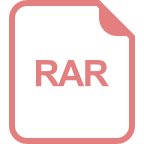
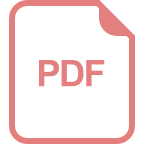
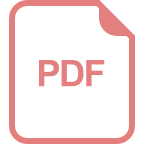
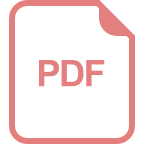
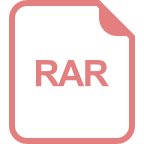
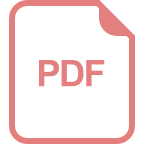
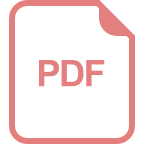
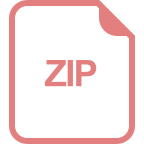
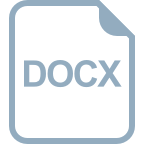