python建立倒排索引表
时间: 2023-06-29 20:20:24 浏览: 107
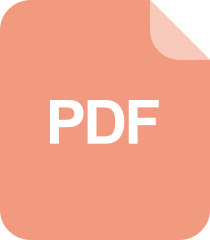
python 实现倒排索引的方法
要建立一个简单的倒排索引表,可以按照以下步骤进行:
1. 读取文本文件,将每个文档中的单词分解成一个个的词项。
2. 对每个词项建立一个包含该词项的文档列表。
3. 对文档列表进行排序,可以按照文档编号或者其他排序方式进行排序。
4. 建立一个词项与文档列表的映射关系表。
以下是一个示例代码:
```
import os
# 读取文本文件,将每个文档中的单词分解成一个个的词项
def get_words(file_path):
with open(file_path, 'r') as f:
return f.read().split()
# 对每个词项建立一个包含该词项的文档列表
def build_index(file_dir):
index = {}
for file_name in os.listdir(file_dir):
file_path = os.path.join(file_dir, file_name)
words = set(get_words(file_path))
for word in words:
if word not in index:
index[word] = []
index[word].append(file_name)
return index
# 对文档列表进行排序
def sort_index(index):
for word in index:
index[word].sort()
# 建立一个词项与文档列表的映射关系表
def build_mapping(index):
mapping = {}
for word in index:
mapping[word] = ','.join(index[word])
return mapping
# 示例
file_dir = './documents'
index = build_index(file_dir)
sort_index(index)
mapping = build_mapping(index)
print(mapping)
```
在上面的示例代码中,`file_dir` 是包含文本文件的文件夹,`build_index` 函数会对每个文本文件建立倒排索引表,`sort_index` 函数会对文档列表进行排序,`build_mapping` 函数会建立一个词项与文档列表的映射关系表。最后,我们可以打印出这个映射关系表,得到每个词项对应的文档列表。
阅读全文
相关推荐
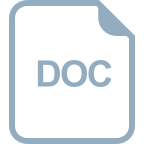

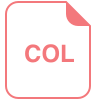
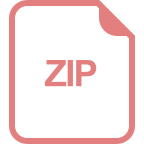
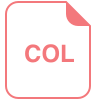
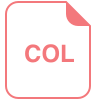
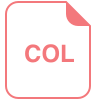
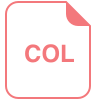
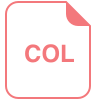
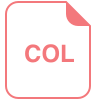
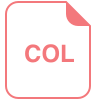
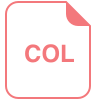
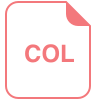
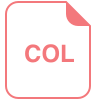
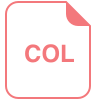
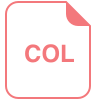