可否距离写一份servlet➕JSP的代码给我作为演示呢
时间: 2024-03-15 08:42:25 浏览: 55
当然可以,以下是一个简单的购物车示例代码(仅供参考):
商品列表页面(productList.jsp):
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>商品列表</title>
</head>
<body>
<h1>商品列表</h1>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>价格</th>
<th>库存</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${products}" var="product">
<tr>
<td>${product.name}</td>
<td>${product.price}</td>
<td>${product.stock}</td>
<td>
<button onclick="addToCart('${product.id}')">添加到购物车</button>
</td>
</tr>
</c:forEach>
</tbody>
</table>
<script>
function addToCart(productId) {
// 将商品信息发送到Servlet,使用Ajax实现异步请求
var xhr = new XMLHttpRequest();
xhr.open('POST', 'cartServlet', true);
xhr.setRequestHeader('Content-type', 'application/x-www-form-urlencoded');
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
alert('添加成功');
}
};
xhr.send('productId=' + productId + '&action=add');
}
</script>
</body>
</html>
```
购物车页面(cart.jsp):
```jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>购物车</title>
</head>
<body>
<h1>购物车</h1>
<table>
<thead>
<tr>
<th>商品名称</th>
<th>价格</th>
<th>数量</th>
<th>小计</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<c:forEach items="${cart.items}" var="item">
<tr>
<td>${item.product.name}</td>
<td>${item.product.price}</td>
<td>${item.quantity}</td>
<td>${item.subtotal}</td>
<td>
<button onclick="removeFromCart('${item.product.id}')">删除</button>
</td>
</tr>
</c:forEach>
<tr>
<td colspan="3">总计:</td>
<td>${cart.total}</td>
<td><button onclick="clearCart()">清空购物车</button></td>
</tr>
</tbody>
</table>
<script>
function removeFromCart(productId) {
// 将商品信息发送到Servlet,使用Ajax实现异步请求
var xhr = new XMLHttpRequest();
xhr.open('POST', 'cartServlet', true);
xhr.setRequestHeader('Content-type', 'application/x-www-form-urlencoded');
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
window.location.reload();
}
};
xhr.send('productId=' + productId + '&action=remove');
}
function clearCart() {
// 将购物车信息发送到Servlet,使用Ajax实现异步请求
var xhr = new XMLHttpRequest();
xhr.open('POST', 'cartServlet', true);
xhr.setRequestHeader('Content-type', 'application/x-www-form-urlencoded');
xhr.onreadystatechange = function() {
if (xhr.readyState === XMLHttpRequest.DONE && xhr.status === 200) {
window.location.reload();
}
};
xhr.send('action=clear');
}
</script>
</body>
</html>
```
Cart类:
```java
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class Cart {
private Map<String, CartItem> items = new HashMap<>();
private BigDecimal total = BigDecimal.ZERO;
public void add(Product product) {
CartItem item = items.get(product.getId());
if (item == null) {
item = new CartItem(product);
items.put(product.getId(), item);
}
item.setQuantity(item.getQuantity() + 1);
item.setSubtotal(item.getProduct().getPrice().multiply(new BigDecimal(item.getQuantity())));
total = total.add(product.getPrice());
}
public void remove(String productId) {
CartItem item = items.get(productId);
if (item == null) {
return;
}
total = total.subtract(item.getSubtotal());
items.remove(productId);
}
public void clear() {
items.clear();
total = BigDecimal.ZERO;
}
public List<CartItem> getItems() {
return new ArrayList<>(items.values());
}
public BigDecimal getTotal() {
return total;
}
public static class CartItem {
private Product product;
private int quantity = 0;
private BigDecimal subtotal = BigDecimal.ZERO;
public CartItem(Product product) {
this.product = product;
}
public Product getProduct() {
return product;
}
public void setProduct(Product product) {
this.product = product;
}
public int getQuantity() {
return quantity;
}
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public BigDecimal getSubtotal() {
return subtotal;
}
public void setSubtotal(BigDecimal subtotal) {
this.subtotal = subtotal;
}
}
}
```
Product类:
```java
import java.math.BigDecimal;
public class Product {
private String id;
private String name;
private BigDecimal price;
private int stock;
public Product(String id, String name, BigDecimal price, int stock) {
this.id = id;
this.name = name;
this.price = price;
this.stock = stock;
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public BigDecimal getPrice() {
return price;
}
public void setPrice(BigDecimal price) {
this.price = price;
}
public int getStock() {
return stock;
}
public void setStock(int stock) {
this.stock = stock;
}
}
```
CartServlet:
```java
import java.io.IOException;
import java.math.BigDecimal;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
@WebServlet("/cartServlet")
public class CartServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
private Map<String, Product> products = new HashMap<>();
private Cart cart = new Cart();
public CartServlet() {
super();
// 初始化测试数据
products.put("1", new Product("1", "商品1", new BigDecimal("100"), 10));
products.put("2", new Product("2", "商品2", new BigDecimal("200"), 5));
products.put("3", new Product("3", "商品3", new BigDecimal("300"), 8));
}
protected void doPost(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
String productId = request.getParameter("productId");
String action = request.getParameter("action");
if ("add".equals(action)) {
Product product = products.get(productId);
cart.add(product);
} else if ("remove".equals(action)) {
cart.remove(productId);
} else if ("clear".equals(action)) {
cart.clear();
}
// 将购物车信息存储到Session中
request.getSession().setAttribute("cart", cart);
// 返回操作结果
response.getWriter().write("ok");
}
protected void doGet(HttpServletRequest request, HttpServletResponse response)
throws ServletException, IOException {
// 获取购物车信息
Cart cart = (Cart) request.getSession().getAttribute("cart");
if (cart == null) {
cart = new Cart();
}
// 获取商品列表
List<Product> productList = List.copyOf(products.values());
// 跳转到商品列表页面
request.setAttribute("products", productList);
request.getRequestDispatcher("productList.jsp").forward(request, response);
}
}
```
以上代码仅供参考,实际应用中还需要进行较多的优化和完善。
阅读全文
相关推荐
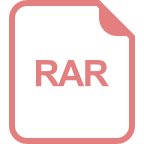
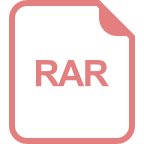
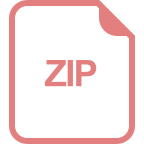
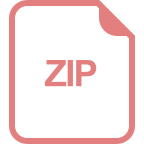
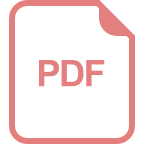
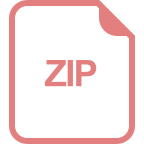
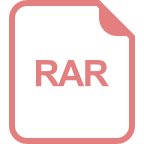
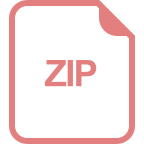
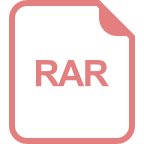
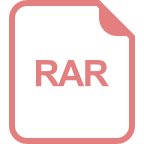
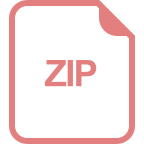
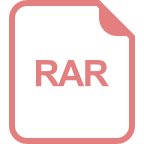
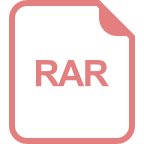
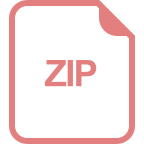
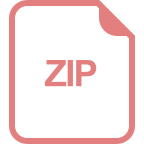
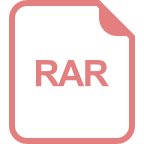
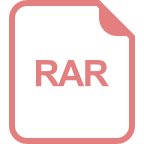