如何用Java做动态爱心
时间: 2024-10-13 08:17:49 浏览: 23
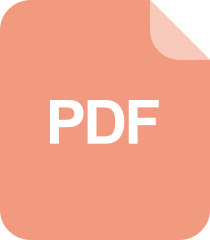
java代码实现动态爱心代码示例
在Java中制作动态爱心形状通常需要使用Swing或JavaFX库来绘制图形,这里我会提供一个简单的JavaFX示例来创建一个旋转的心形动画。首先,你需要导入JavaFX相关的包,并且了解如何使用`Path`、`Rotate`和`Timeline`。
```java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.layout.StackPane;
import javafx.scene.paint.Color;
import javafx.scene.shape.Path;
import javafx.scene.shape.Shape;
import javafx.stage.Stage;
import javafx.animation.AnimationTimer;
import javafx.animation.Rotate;
public class DynamicHeart extends Application {
private Shape heartShape;
private double rotationAngle = 0;
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
heartShape = createHeartShape();
Scene scene = new Scene(new StackPane(heartShape), 400, 400, Color.TRANSPARENT);
// 创建定时器,每秒旋转心形
AnimationTimer timer = new AnimationTimer() {
@Override
public void handle(long now) {
rotationAngle += 5; // 每次增加5度
heartShape.setTransform(new Rotate(rotationAngle, heartShape.getBoundsInLocal().getWidth() / 2, heartShape.getBoundsInLocal().getHeight() / 2));
if (rotationAngle >= 360) { // 当达到一圈时,重置角度
rotationAngle = 0;
}
}
};
timer.start();
primaryStage.setScene(scene);
primaryStage.show();
}
private Shape createHeartShape() {
double width = 200;
double height = 150;
Path path = new Path();
path.setFill(Color.RED);
path.moveTo(-width * 0.87, -height);
path.lineTo(-width * 0.5, -height * 1.15);
path.lineTo(0, -height * 0.9);
path.lineTo(width * 0.5, -height * 1.15);
path.lineTo(width * 0.87, -height);
path.lineTo(width * 0.5, -height * 0.9);
path.lineTo(0, -height * 0.65);
path.lineTo(-width * 0.5, -height * 0.9);
path.close();
return path;
}
}
```
阅读全文
相关推荐
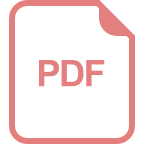














