java随机读取MP3音频并播放
时间: 2023-10-18 18:19:07 浏览: 156
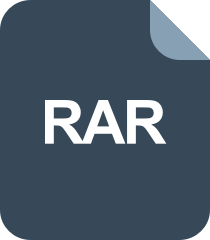
Suijishu.rar_随机播放
要实现Java随机读取MP3音频并播放,你可以使用Java的RandomAccessFile类和JavaFX的MediaPlayer类。以下是实现的步骤:
1. 首先,导入JavaFX库并创建一个JavaFX应用程序。
2. 然后,使用Java的RandomAccessFile类打开MP3文件并跳转到随机位置。
3. 接下来,将随机位置的数据读入一个字节数组中。
4. 将字节数组转换为JavaFX的Media对象。
5. 创建一个MediaPlayer对象,并将Media对象传递给它。
6. 最后,播放音频。
以下是一个示例代码:
```java
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import javafx.application.Application;
import javafx.scene.media.Media;
import javafx.scene.media.MediaPlayer;
import javafx.stage.Stage;
public class RandomMP3Player extends Application {
private static final String FILENAME = "music.mp3";
private static final int BUFFER_SIZE = 1024;
@Override
public void start(Stage primaryStage) throws Exception {
// 创建MediaPlayer对象
MediaPlayer mediaPlayer = new MediaPlayer(null);
// 播放音频
mediaPlayer.play();
// 随机读取音频并播放
while (true) {
// 随机生成一个位置
long position = (long) (Math.random() * (new File(FILENAME).length() - BUFFER_SIZE));
// 读取随机位置的数据
byte[] mp3Bytes = readMP3(FILENAME, position);
// 将字节数组转换为JavaFX的Media对象
Media media = new Media("data:audio/mp3;base64," +
java.util.Base64.getEncoder().encodeToString(mp3Bytes));
// 设置MediaPlayer的Media对象并播放音频
mediaPlayer.stop();
mediaPlayer = new MediaPlayer(media);
mediaPlayer.play();
// 等待1秒钟
Thread.sleep(1000);
}
}
private byte[] readMP3(String filename, long position) throws IOException {
File file = new File(filename);
ByteBuffer buffer = ByteBuffer.allocate(BUFFER_SIZE);
try (RandomAccessFile raFile = new RandomAccessFile(file, "r");
FileChannel fileChannel = raFile.getChannel()) {
fileChannel.position(position);
int bytesRead = fileChannel.read(buffer);
buffer.flip();
byte[] mp3Bytes = new byte[bytesRead];
buffer.get(mp3Bytes, 0, bytesRead);
return mp3Bytes;
}
}
public static void main(String[] args) {
launch(args);
}
}
```
在此示例中,我们使用一个循环来随机读取MP3文件并播放音频。在循环中,我们使用Math.random()方法生成一个随机位置,并使用readMP3()方法读取随机位置的数据。然后,我们将字节数组转换为Base64编码的字符串,并将其传递给JavaFX的Media对象。最后,我们设置MediaPlayer的Media对象并播放音频。请注意,我们使用Thread.sleep()方法等待1秒钟,以便在播放下一个音频之前暂停。
阅读全文
相关推荐
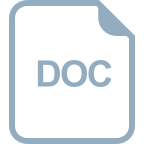
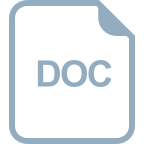

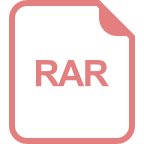
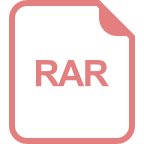
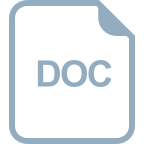
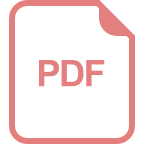
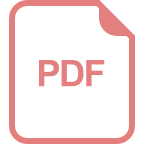
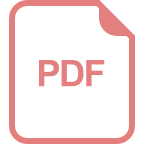
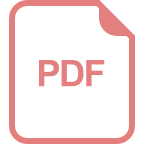
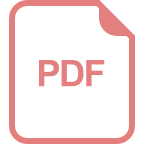
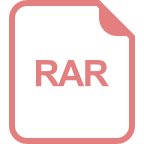
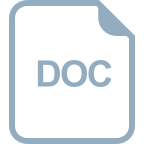
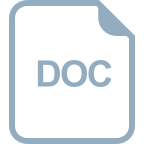
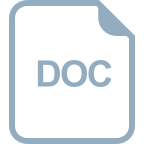
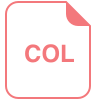
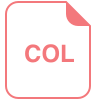
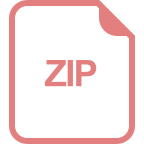