写一串单链表通讯录管理系统的C语言代码
时间: 2023-11-29 19:05:41 浏览: 81
下面是一个简单的单链表通讯录管理系统的C语言代码,包含添加联系人、删除联系人、查找联系人、显示所有联系人等功能:
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef struct Contact {
char name[50];
char phone[20];
struct Contact *next;
} Contact;
Contact *head = NULL;
void addContact() {
Contact *newContact = malloc(sizeof(Contact));
printf("Enter name: ");
scanf("%s", newContact->name);
printf("Enter phone number: ");
scanf("%s", newContact->phone);
newContact->next = NULL;
if (head == NULL) {
head = newContact;
} else {
Contact *p = head;
while (p->next != NULL) {
p = p->next;
}
p->next = newContact;
}
printf("Contact added successfully\n");
}
void deleteContact() {
char name[50];
printf("Enter name to delete: ");
scanf("%s", name);
if (head == NULL) {
printf("Contact not found\n");
} else if (strcmp(head->name, name) == 0) {
Contact *temp = head;
head = head->next;
free(temp);
printf("Contact deleted successfully\n");
} else {
Contact *p = head;
while (p->next != NULL && strcmp(p->next->name, name) != 0) {
p = p->next;
}
if (p->next == NULL) {
printf("Contact not found\n");
} else {
Contact *temp = p->next;
p->next = temp->next;
free(temp);
printf("Contact deleted successfully\n");
}
}
}
void searchContact() {
char name[50];
printf("Enter name to search: ");
scanf("%s", name);
Contact *p = head;
while (p != NULL && strcmp(p->name, name) != 0) {
p = p->next;
}
if (p == NULL) {
printf("Contact not found\n");
} else {
printf("Name: %s\nPhone: %s\n", p->name, p->phone);
}
}
void displayContacts() {
printf("Contacts:\n");
Contact *p = head;
while (p != NULL) {
printf("Name: %s\nPhone: %s\n", p->name, p->phone);
p = p->next;
}
}
int main() {
int choice;
while (1) {
printf("\nMenu:\n");
printf("1. Add contact\n");
printf("2. Delete contact\n");
printf("3. Search contact\n");
printf("4. Display all contacts\n");
printf("5. Exit\n");
printf("Enter your choice: ");
scanf("%d", &choice);
switch (choice) {
case 1:
addContact();
break;
case 2:
deleteContact();
break;
case 3:
searchContact();
break;
case 4:
displayContacts();
break;
case 5:
exit(0);
default:
printf("Invalid choice\n");
}
}
return 0;
}
```
该代码使用了无限循环,直到用户选择退出才结束。在每次循环中,根据用户的选择执行相应的操作。
阅读全文
相关推荐






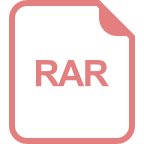
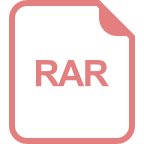
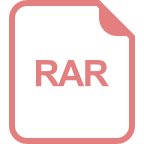
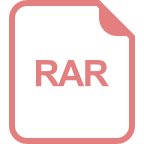




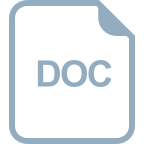