for_each处理函数传参
时间: 2023-07-30 22:09:07 浏览: 102
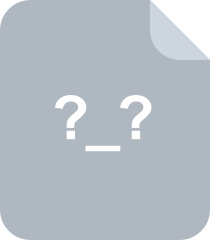
for_each.cpp
`for_each`是STL中的一个算法,用于对一个区间内的元素进行操作。它接受三个参数:待处理区间的起始迭代器、待处理区间的结束迭代器和一个处理函数。
处理函数可以是一个函数指针、函数对象或者lambda表达式。它的参数类型取决于待处理区间内元素的类型。在调用处理函数时,`for_each`会将当前元素作为参数传递给处理函数。如果处理函数需要额外的参数,可以通过lambda表达式或者bind函数来实现。例如:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
#include <functional>
void print(int x) {
std::cout << x << ' ';
}
int main() {
std::vector<int> v = {1, 2, 3, 4, 5};
std::for_each(v.begin(), v.end(), print); // 输出: 1 2 3 4 5
int factor = 2;
auto multiply = [&](int x) { // 使用lambda表达式传递额外参数
std::cout << x * factor << ' ';
};
std::for_each(v.begin(), v.end(), multiply); // 输出: 2 4 6 8 10
auto add = std::bind(std::plus<int>(), std::placeholders::_1, 3); // 使用bind函数传递额外参数
std::for_each(v.begin(), v.end(), add); // 输出: 4 5 6 7 8
return 0;
}
```
上面的代码演示了如何使用`for_each`函数传递不同类型的处理函数和额外参数。注意,当处理函数是lambda表达式或者绑定函数时,需要使用auto关键字声明处理函数。
阅读全文
相关推荐
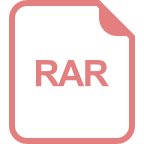
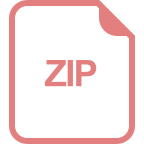















