存储一个家族的族谱,并提供查询和修改。要求刚开始的族谱里至少有10人,能够查询某个指定人物的双亲和他所有的子孙,新增子孙的插入,指定人物由键盘输入。测试上述所有功能。要求使用树相关知识实现。
时间: 2024-03-06 09:47:41 浏览: 21
一个家族的族谱可以通过二叉树来表示。在二叉树中,每个节点都有一个指向其父节点的指针和一个指向其左右子节点的指针。我们可以定义一个Person类来表示家族中的每个人,每个Person对象包含以下属性:
- name:姓名
- gender:性别
- parent:父亲节点
- leftChild:左子节点
- rightChild:右子节点
然后,我们可以通过构建Person对象之间的二叉树来表示家族家谱。具体实现过程可以参考以下Java代码:
```java
public class Person {
private String name;
private String gender;
private Person parent;
private Person leftChild;
private Person rightChild;
// 构造函数
public Person(String name, String gender) {
this.name = name;
this.gender = gender;
this.parent = null;
this.leftChild = null;
this.rightChild = null;
}
// 添加子女节点
public void addChild(Person child) {
child.parent = this;
if (this.leftChild == null) {
this.leftChild = child;
} else if (this.rightChild == null) {
this.rightChild = child;
} else {
System.out.println("This person already has two children.");
}
}
// 获取父亲节点
public Person getParent() {
return this.parent;
}
// 获取左子节点
public Person getLeftChild() {
return this.leftChild;
}
// 获取右子节点
public Person getRightChild() {
return this.rightChild;
}
// 获取姓名
public String getName() {
return this.name;
}
// 获取性别
public String getGender() {
return this.gender;
}
}
```
接下来,我们可以定义一个FamilyTree类来表示家族的族谱。FamilyTree类包含以下方法:
- addPerson:添加一个Person对象到族谱中
- searchParent:查询指定人物的父亲节点
- searchChildren:查询指定人物的所有子孙节点
- insertChildren:向指定人物添加一个子孙节点
- printTree:打印整个族谱
具体实现过程可以参考以下Java代码:
```java
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.Queue;
import java.util.Scanner;
public class FamilyTree {
private Person root;
private ArrayList<Person> people;
// 构造函数
public FamilyTree() {
this.root = null;
this.people = new ArrayList<Person>();
}
// 添加一个Person对象到族谱中
public void addPerson(Person person) {
if (this.root == null) {
this.root = person;
} else {
for (Person p : this.people) {
if (p.getLeftChild() == null) {
p.addChild(person);
break;
} else if (p.getRightChild() == null) {
p.addChild(person);
break;
}
}
}
this.people.add(person);
}
// 查询指定人物的父亲节点
public Person searchParent(String name) {
for (Person p : this.people) {
if (p.getName().equals(name)) {
return p.getParent();
}
}
return null;
}
// 查询指定人物的所有子孙节点
public ArrayList<Person> searchChildren(String name) {
ArrayList<Person> children = new ArrayList<Person>();
for (Person p : this.people) {
if (p.getName().equals(name)) {
Queue<Person> queue = new LinkedList<Person>();
queue.offer(p);
while (!queue.isEmpty()) {
Person node = queue.poll();
if (node.getLeftChild() != null) {
queue.offer(node.getLeftChild());
}
if (node.getRightChild() != null) {
queue.offer(node.getRightChild());
}
children.add(node);
}
break;
}
}
return children;
}
// 向指定人物添加一个子孙节点
public void insertChildren(String name, Person child) {
for (Person p : this.people) {
if (p.getName().equals(name)) {
p.addChild(child);
this.people.add(child);
break;
}
}
}
// 打印整个族谱
public void printTree() {
if (this.root == null) {
System.out.println("The family tree is empty.");
} else {
Queue<Person> queue = new LinkedList<Person>();
queue.offer(this.root);
while (!queue.isEmpty()) {
int levelSize = queue.size();
for (int i = 0; i < levelSize; i++) {
Person node = queue.poll();
System.out.print(node.getName() + " ");
if (node.getLeftChild() != null) {
queue.offer(node.getLeftChild());
}
if (node.getRightChild() != null) {
queue.offer(node.getRightChild());
}
}
System.out.println();
}
}
}
// 测试函数
public static void main(String[] args) {
FamilyTree tree = new FamilyTree();
// 初始化族谱
Person grandpa = new Person("Grandpa", "Male");
Person grandma = new Person("Grandma", "Female");
Person dad = new Person("Dad", "Male");
Person uncle1 = new Person("Uncle1", "Male");
Person uncle2 = new Person("Uncle2", "Male");
Person son1 = new Person("Son1", "Male");
Person son2 = new Person("Son2", "Male");
Person son3 = new Person("Son3", "Male");
Person daughter = new Person("Daughter", "Female");
tree.addPerson(grandpa);
tree.addPerson(grandma);
tree.addPerson(dad);
tree.addPerson(uncle1);
tree.addPerson(uncle2);
tree.addPerson(son1);
tree.addPerson(son2);
tree.addPerson(son3);
tree.addPerson(daughter);
grandpa.addChild(dad);
grandpa.addChild(uncle1);
grandpa.addChild(uncle2);
dad.addChild(son1);
dad.addChild(son2);
dad.addChild(son3);
dad.addChild(daughter);
// 测试查询父亲节点
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter a person's name: ");
String name = scanner.nextLine();
Person parent = tree.searchParent(name);
if (parent == null) {
System.out.println("This person is not in the family tree.");
} else {
System.out.println(parent.getName() + " is " + name + "'s parent.");
}
// 测试查询所有子孙节点
System.out.print("Please enter a person's name: ");
name = scanner.nextLine();
ArrayList<Person> children = tree.searchChildren(name);
if (children.size() == 0) {
System.out.println("This person is not in the family tree.");
} else {
System.out.println(name + "'s children and grandchildren are:");
for (Person p : children) {
System.out.println(p.getName());
}
}
// 测试添加子孙节点
System.out.print("Please enter a person's name: ");
name = scanner.nextLine();
System.out.print("Please enter the name of the new child: ");
String childName = scanner.nextLine();
System.out.print("Please enter the gender of the new child (Male/Female): ");
String gender = scanner.nextLine();
Person child = new Person(childName, gender);
tree.insertChildren(name, child);
// 打印整个族谱
tree.printTree();
}
}
```
这样,我们就可以通过FamilyTree类来存储一个家族的族谱,并提供查询和修改功能。
相关推荐
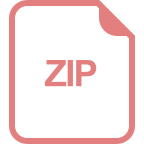
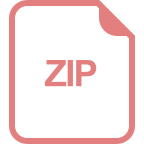

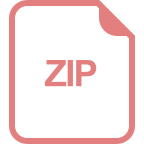
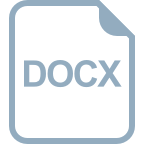
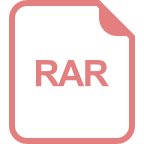
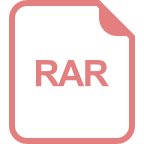
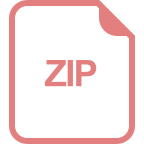
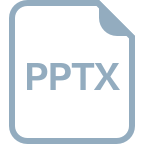
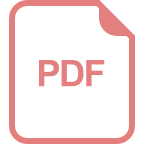