c语言 获取linuxipv6路由表
时间: 2023-09-23 15:13:18 浏览: 151
可以使用Socket编程来获取Linux的IPv6路由表。下面是一个使用C语言编写的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <sys/socket.h>
#include <netinet/in.h>
#include <netinet/ip6.h>
#include <netinet/icmp6.h>
#include <arpa/inet.h>
#include <net/if.h>
#include <net/route.h>
#define BUF_SIZE 4096
int main() {
int sock;
struct rtmsg *route_entry;
struct nlmsghdr *nl_header;
struct rtattr *rt_attribute;
char buffer[BUF_SIZE];
// 创建socket
sock = socket(AF_NETLINK, SOCK_RAW, NETLINK_ROUTE);
if (sock < 0) {
perror("socket");
exit(1);
}
// 初始化请求消息
memset(buffer, 0, BUF_SIZE);
nl_header = (struct nlmsghdr *)buffer;
nl_header->nlmsg_len = NLMSG_LENGTH(sizeof(struct rtmsg));
nl_header->nlmsg_type = RTM_GETROUTE;
nl_header->nlmsg_flags = NLM_F_DUMP | NLM_F_REQUEST;
nl_header->nlmsg_seq = 1;
nl_header->nlmsg_pid = getpid();
// 设置请求消息的路由属性
rt_attribute = (struct rtattr *)NLMSG_DATA(nl_header);
rt_attribute->rta_type = RTA_DST;
rt_attribute->rta_len = RTA_LENGTH(sizeof(struct in6_addr));
inet_pton(AF_INET6, "2001:db8::/32", RTA_DATA(rt_attribute));
// 发送请求消息
if (send(sock, nl_header, nl_header->nlmsg_len, 0) < 0) {
perror("send");
exit(1);
}
// 接收并处理回复消息
while (1) {
int len = recv(sock, buffer, BUF_SIZE, 0);
if (len < 0) {
perror("recv");
exit(1);
}
for (nl_header = (struct nlmsghdr *)buffer; NLMSG_OK(nl_header, len); nl_header = NLMSG_NEXT(nl_header, len)) {
if (nl_header->nlmsg_type == NLMSG_DONE) {
break;
}
route_entry = (struct rtmsg *)NLMSG_DATA(nl_header);
rt_attribute = (struct rtattr *)RTM_RTA(route_entry);
int rt_attr_len = RTM_PAYLOAD(nl_header);
for (; RTA_OK(rt_attribute, rt_attr_len); rt_attribute = RTA_NEXT(rt_attribute, rt_attr_len)) {
if (rt_attribute->rta_type == RTA_DST) {
struct in6_addr *addr = (struct in6_addr *)RTA_DATA(rt_attribute);
char ip_str[INET6_ADDRSTRLEN];
inet_ntop(AF_INET6, addr, ip_str, sizeof(ip_str));
printf("Route: %s\n", ip_str);
}
}
}
}
// 关闭socket
close(sock);
return 0;
}
```
注意:需要在代码中修改`inet_pton(AF_INET6, "2001:db8::/32", RTA_DATA(rt_attribute))`这一行,将`"2001:db8::/32"`改为你想要查询的IPv6网段。
这段代码使用Netlink套接字接口向内核发送RTM_GETROUTE消息,并解析内核的回复,将获取到的IPv6路由表打印出来。请确保以root权限运行代码,因为获取路由表需要特权访问。
希望对你有帮助!如果有任何疑问,请随时提问。
阅读全文
相关推荐












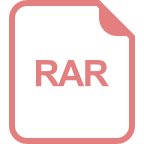
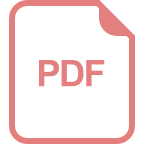