Promise实现原理,给一份实现代码
时间: 2023-08-02 09:16:09 浏览: 80
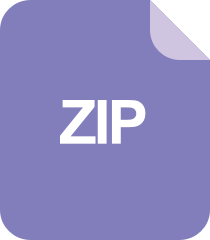
promise实现原理
Promise 是一种异步编程的解决方案,其本质上是一个状态机,包含三种状态:pending、fulfilled 和 rejected。
以下是 Promise 的基本实现代码:
```javascript
class Promise {
constructor(executor) {
this.state = 'pending';
this.value = undefined;
this.reason = undefined;
this.onFulfilledCallbacks = [];
this.onRejectedCallbacks = [];
const resolve = (value) => {
if (this.state === 'pending') {
this.state = 'fulfilled';
this.value = value;
this.onFulfilledCallbacks.forEach((callback) => callback());
}
};
const reject = (reason) => {
if (this.state === 'pending') {
this.state = 'rejected';
this.reason = reason;
this.onRejectedCallbacks.forEach((callback) => callback());
}
};
try {
executor(resolve, reject);
} catch (error) {
reject(error);
}
}
then(onFulfilled, onRejected) {
onFulfilled = typeof onFulfilled === 'function' ? onFulfilled : (value) => value;
onRejected = typeof onRejected === 'function' ? onRejected : (reason) => { throw reason };
const promise2 = new Promise((resolve, reject) => {
if (this.state === 'fulfilled') {
setTimeout(() => {
try {
const x = onFulfilled(this.value);
resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
} else if (this.state === 'rejected') {
setTimeout(() => {
try {
const x = onRejected(this.reason);
resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
} else {
this.onFulfilledCallbacks.push(() => {
setTimeout(() => {
try {
const x = onFulfilled(this.value);
resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
});
this.onRejectedCallbacks.push(() => {
setTimeout(() => {
try {
const x = onRejected(this.reason);
resolvePromise(promise2, x, resolve, reject);
} catch (error) {
reject(error);
}
}, 0);
});
}
});
return promise2;
}
catch(onRejected) {
return this.then(null, onRejected);
}
}
const resolvePromise = (promise2, x, resolve, reject) => {
if (promise2 === x) {
reject(new TypeError('Chaining cycle detected for promise'));
}
let called = false;
if (x !== null && (typeof x === 'object' || typeof x === 'function')) {
try {
const then = x.then;
if (typeof then === 'function') {
then.call(
x,
(y) => {
if (called) return;
called = true;
resolvePromise(promise2, y, resolve, reject);
},
(r) => {
if (called) return;
called = true;
reject(r);
}
);
} else {
resolve(x);
}
} catch (error) {
if (called) return;
called = true;
reject(error);
}
} else {
resolve(x);
}
};
```
这个实现代码中,我们首先定义了一个 Promise 类,其构造函数接受一个 executor 函数,如果在 executor 函数中调用了 resolve 方法,则 Promise 的状态会变为 fulfilled,如果调用了 reject 方法,则 Promise 的状态会变为 rejected。
在 then 方法中,我们首先对 onFulfilled 和 onRejected 进行了类型判断,然后根据当前 Promise 的状态,分别执行对应的回调函数。如果当前 Promise 的状态是 pending,我们则将回调函数存储到 onFulfilledCallbacks 或 onRejectedCallbacks 中,等待 Promise 的状态发生变化后再执行。
在 resolvePromise 方法中,我们首先判断 x 是否等于 promise2,如果是则说明出现了循环引用的情况,直接抛出错误。然后我们判断 x 是否为对象或函数,如果是,则尝试获取其 then 方法。如果获取到了,则调用 then 方法,并将 resolve 和 reject 方法作为参数传递给 then 方法。如果 then 方法返回值仍然是一个 Promise,则递归调用 resolvePromise 方法,直到返回值不为 Promise 为止。否则,直接将返回值作为 Promise 的结果传递给下一个 then 方法。如果 x 不是对象或函数,则直接将其传递给下一个 then 方法。
阅读全文
相关推荐
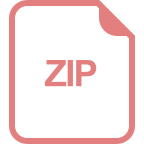
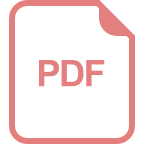
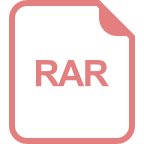
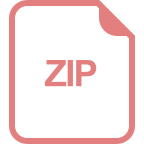
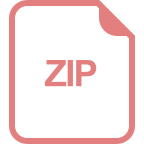
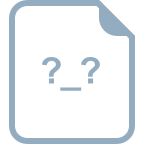
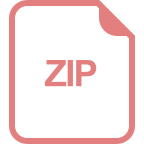
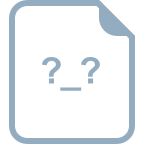
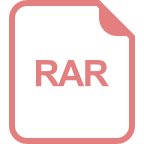
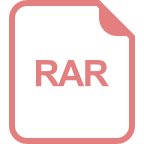
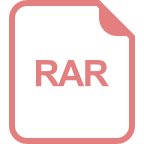
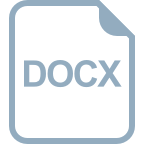
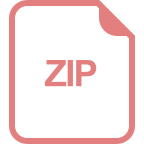
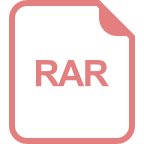
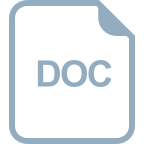
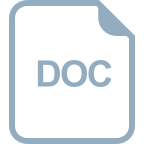