python进阶版Cucumber示例
时间: 2023-06-28 16:16:07 浏览: 246
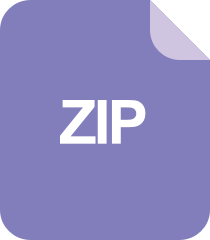
cucumber-example
以下是一个简单的Python进阶版Cucumber示例:
假设我们要测试一个简单的计算器程序,它能够执行加、减、乘、除四种基本运算。我们可以使用Python的unittest模块来编写测试用例,并使用Behave框架来编写BDD测试。
首先,我们需要安装Behave框架:
```
pip install behave
```
然后,我们可以编写.feature文件,描述我们要测试的场景:
```
Feature: Calculator
Scenario: Addition
Given I have entered 10 into the calculator
And I have entered 5 into the calculator
When I press add
Then the result should be 15 on the screen
Scenario: Subtraction
Given I have entered 10 into the calculator
And I have entered 5 into the calculator
When I press subtract
Then the result should be 5 on the screen
Scenario: Multiplication
Given I have entered 10 into the calculator
And I have entered 5 into the calculator
When I press multiply
Then the result should be 50 on the screen
Scenario: Division
Given I have entered 10 into the calculator
And I have entered 5 into the calculator
When I press divide
Then the result should be 2 on the screen
```
接下来,我们可以编写.steps文件,实现.feature文件中描述的步骤:
```python
from behave import given, when, then
from calculator import Calculator
@given('I have entered {num:d} into the calculator')
def step_impl(context, num):
context.calc = Calculator()
context.calc.enter(num)
@when('I press add')
def step_impl(context):
context.calc.add()
@when('I press subtract')
def step_impl(context):
context.calc.subtract()
@when('I press multiply')
def step_impl(context):
context.calc.multiply()
@when('I press divide')
def step_impl(context):
context.calc.divide()
@then('the result should be {result:d} on the screen')
def step_impl(context, result):
assert context.calc.result == result
```
最后,我们可以编写一个Calculator类来实现计算器的基本功能:
```python
class Calculator:
def __init__(self):
self.result = 0
def enter(self, num):
self.result = num
def add(self):
self.result += self.current
def subtract(self):
self.result -= self.current
def multiply(self):
self.result *= self.current
def divide(self):
self.result /= self.current
```
现在,我们可以在终端中执行以下命令来运行测试:
```
behave calculator.feature
```
这将运行.feature文件中描述的所有场景,并报告测试结果。
这是一个简单的Python进阶版Cucumber示例,演示了如何使用Behave框架编写BDD测试用例。
阅读全文
相关推荐
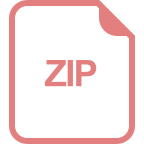
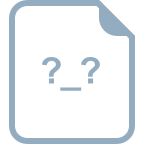

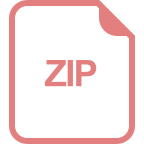
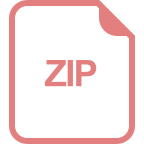
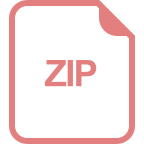
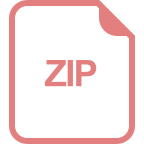
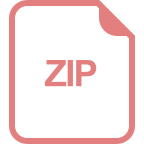
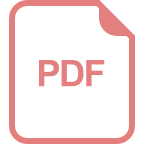
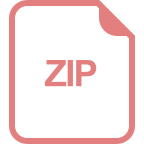
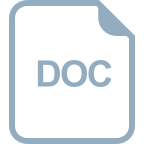
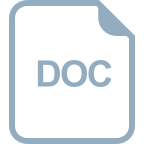
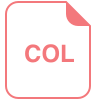
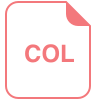
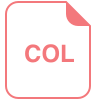
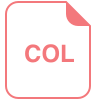
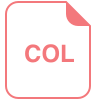
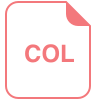