写一个Node.js程序,检查当前目录下是否存在data文件夹。如果存在,打印出文件夹中的所有文件名;如果不存在,创建一个data文件夹。使用fs模块和path模块中的函数实现。
时间: 2024-05-14 15:15:07 浏览: 40
```javascript
const fs = require('fs');
const path = require('path');
const dirPath = path.join(__dirname, 'data');
if (!fs.existsSync(dirPath)) {
fs.mkdirSync(dirPath);
console.log('data文件夹已创建');
} else {
console.log('data文件夹中的文件有:');
const files = fs.readdirSync(dirPath);
files.forEach(file => console.log(file));
}
```
相关问题
2.写一个Node.js程序,检查当前目录下是否存在data文件夹。如果存在,打印出文件夹中的所有文件名;如果不存在,创建一个data文件夹。使用fs模块和path模块中的函数实现。 readdir
const fs = require('fs');
const path = require('path');
const dataFolderPath = path.join(__dirname, 'data');
if (fs.existsSync(dataFolderPath)) {
// 如果data文件夹存在,打印文件名
fs.readdir(dataFolderPath, (err, files) => {
if (err) throw err;
console.log('data文件夹中的文件有:');
files.forEach(file => {
console.log(file);
});
});
} else {
// 如果data文件夹不存在,创建文件夹
fs.mkdir(dataFolderPath, (err) => {
if (err) throw err;
console.log('data文件夹创建成功');
});
}
写一个Node.js程序,读取data文件夹中的所有.txt文件,将它们的内容合并到一个文件combined.txt中。使用fs模块和path模块中的函数实现。
```
const fs = require('fs');
const path = require('path');
const dataFolderPath = path.join(__dirname, 'data');
fs.readdir(dataFolderPath, (err, files) => {
if (err) {
console.error(err);
return;
}
const txtFiles = files.filter(file => path.extname(file) === '.txt');
const combinedFilePath = path.join(__dirname, 'combined.txt');
fs.writeFile(combinedFilePath, '', err => {
if (err) {
console.error(err);
return;
}
txtFiles.forEach(txtFile => {
const txtFilePath = path.join(dataFolderPath, txtFile);
fs.readFile(txtFilePath, 'utf8', (err, data) => {
if (err) {
console.error(err);
return;
}
fs.appendFile(combinedFilePath, data, err => {
if (err) {
console.error(err);
return;
}
});
});
});
});
});
```
代码说明:
1. 首先使用fs模块的readdir函数读取data文件夹中的所有文件名。
2. 使用path模块的extname函数过滤出所有扩展名为.txt的文件。
3. 指定合并后的文件路径为当前文件夹下的combined.txt。
4. 使用fs模块的writeFile函数创建空的合并文件。
5. 对于每个txt文件,使用fs模块的readFile函数读取其内容,并使用fs模块的appendFile函数将其追加到合并文件中。注意,使用appendFile而不是writeFile是因为我们需要将多个文件的内容追加到同一个文件中。
阅读全文
相关推荐
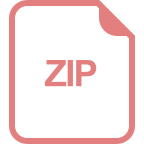
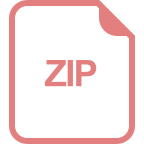
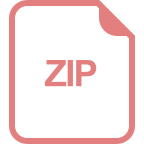
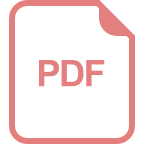
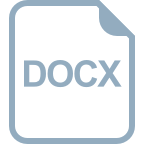
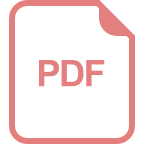
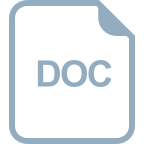
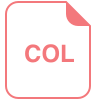
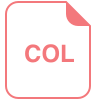
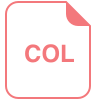
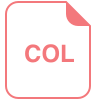
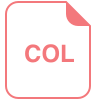



