c++中 图该如何通过目录初始化
时间: 2024-03-20 12:44:38 浏览: 38
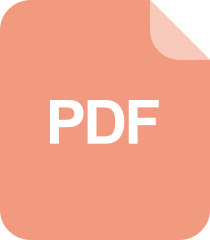
c++ 子类构造函数初始化及父类构造初始化的使用
如果您的图是通过目录(文件夹)中的文件来初始化的话,您可以按照以下步骤来实现:
1. 首先,您需要使用C++标准库中的dirent.h头文件中的函数opendir()、readdir()和closedir()来遍历目录中的文件。具体的代码实现可以参考如下:
```c++
#include <dirent.h>
#include <iostream>
#include <string>
using namespace std;
int main() {
DIR *dir;
struct dirent *ent;
string path = "your directory path";
if ((dir = opendir(path.c_str())) != NULL) {
while ((ent = readdir(dir)) != NULL) {
if (ent->d_type == DT_REG) { //只处理普通文件
cout << ent->d_name << endl; //输出文件名
}
}
closedir(dir);
} else {
perror("");
return EXIT_FAILURE;
}
return EXIT_SUCCESS;
}
```
2. 接下来,您需要根据目录中的文件来建立图。假设您的目录中的文件表示图中的节点,文件名表示节点的编号,文件内容表示节点所连接的其他节点编号。具体的代码实现可以参考如下:
```c++
#include <dirent.h>
#include <fstream>
#include <iostream>
#include <string>
#include <vector>
using namespace std;
int main() {
DIR *dir;
struct dirent *ent;
string path = "your directory path";
if ((dir = opendir(path.c_str())) != NULL) {
while ((ent = readdir(dir)) != NULL) {
if (ent->d_type == DT_REG) { //只处理普通文件
string filename = ent->d_name;
int node = stoi(filename); //节点编号
vector<int> edges; //节点所连接的其他节点编号
ifstream ifs(path + "/" + filename);
int edge;
while (ifs >> edge) {
edges.push_back(edge);
}
ifs.close();
//在图中添加节点以及节点之间的边
}
}
closedir(dir);
} else {
perror("");
return EXIT_FAILURE;
}
return EXIT_SUCCESS;
}
```
需要注意的是,上述代码中的“在图中添加节点以及节点之间的边”的部分需要根据您所使用的图的表示方式进行相应的修改。
阅读全文
相关推荐
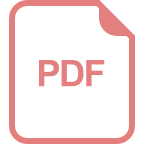
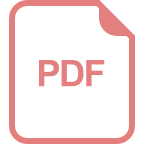
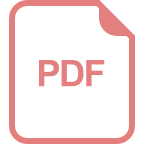
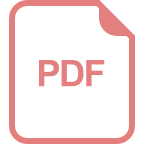
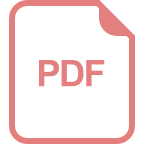
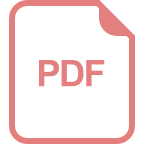
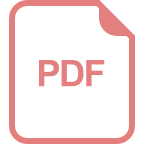
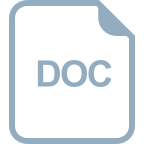
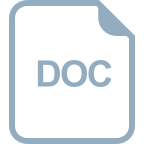
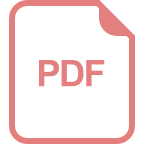
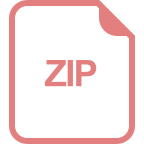
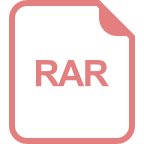
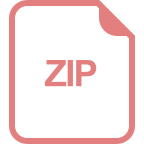