继续
时间: 2024-02-20 08:01:41 浏览: 6
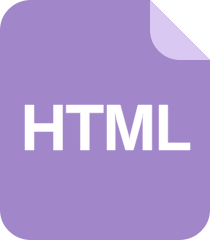
继续游戏
以下是使用LZW算法解压缩图片的Python代码示例:
```python
import struct
def lzw_decompress(data):
# 初始化字典
dictionary = {i: chr(i) for i in range(256)}
next_code = 256
# 初始化输入码流和缓冲区
input_data = struct.unpack('H' * (len(data) // 2), data)
buffer = dictionary[input_data[0]]
# LZW算法解压缩
output = [buffer]
for code in input_data[1:]:
if code in dictionary:
entry = dictionary[code]
elif code == next_code:
entry = buffer + buffer[0]
else:
raise ValueError(f'Invalid LZW code: {code}')
output.append(entry)
dictionary[next_code] = buffer + entry[0]
next_code += 1
buffer = entry
# 将解压缩后的数据打包成二进制文件
decompressed_data = ''.join(output).encode('latin1')
return decompressed_data
# 读取压缩后的数据文件
with open('image_compressed.lzw', 'rb') as f:
compressed_data = f.read()
# 解压缩数据
decompressed_data = lzw_decompress(compressed_data)
# 将解压缩后的数据写入文件
with open('image_decompressed.bmp', 'wb') as f:
f.write(decompressed_data)
```
这段代码将读取名为"image_compressed.lzw"的压缩数据文件,使用LZW算法进行解压缩,并将解压缩后的数据写入名为"image_decompressed.bmp"的文件中。
需要注意的是,这段代码解压缩后的数据是一个字符串,需要根据具体的图片格式进行相应的处理才能得到正确的图片数据。在这个示例中,我们将解压缩后的字符串直接写入了一个名为"image_decompressed.bmp"的文件中,这个文件应该是一个有效的BMP格式图片文件。
阅读全文
相关推荐
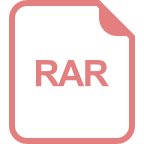
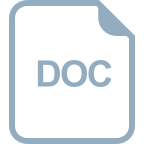