C语言Linux与stm32串口通讯
时间: 2023-07-30 13:10:38 浏览: 147
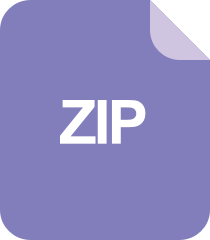
stm32串口通信
在C语言中,可以使用串口通讯协议来实现Linux与stm32之间的通讯。
首先,需要在Linux中配置串口,可以使用以下命令:
```
sudo stty -F /dev/ttyUSB0 115200 cs8 -cstopb -parenb
```
其中,`/dev/ttyUSB0`是串口设备的路径,`115200`是波特率,`cs8`表示8位数据位,`-cstopb`表示1位停止位,`-parenb`表示无校验位。
然后,在C语言中,可以使用串口通讯库,如`termios`库,来进行串口通讯。
以下是一个简单的示例代码:
```c
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
int main()
{
int fd;
struct termios options;
char buffer[255];
// 打开串口设备
fd = open("/dev/ttyUSB0", O_RDWR | O_NOCTTY);
if (fd == -1) {
printf("Open serial port failed!\n");
return -1;
}
// 配置串口
memset(&options, 0, sizeof(options));
cfsetispeed(&options, B115200);
cfsetospeed(&options, B115200);
options.c_cflag |= (CLOCAL | CREAD);
options.c_cflag &= ~PARENB;
options.c_cflag &= ~CSTOPB;
options.c_cflag &= ~CSIZE;
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG);
options.c_iflag &= ~(IXON | IXOFF | IXANY);
options.c_iflag &= ~(INLCR | IGNCR | ICRNL);
options.c_oflag &= ~(ONLCR | OCRNL);
options.c_cc[VTIME] = 0;
options.c_cc[VMIN] = 1;
tcsetattr(fd, TCSANOW, &options);
// 发送数据
write(fd, "Hello, STM32!", 14);
// 接收数据
memset(buffer, 0, sizeof(buffer));
read(fd, buffer, sizeof(buffer));
printf("Received: %s\n", buffer);
// 关闭串口设备
close(fd);
return 0;
}
```
在上述示例代码中,首先使用`open`函数打开串口设备,然后使用`tcsetattr`函数配置串口参数,包括波特率、数据位、停止位、校验位等等。接着,使用`write`函数发送数据,使用`read`函数接收数据。最后,使用`close`函数关闭串口设备。
需要注意的是,在Linux中,串口设备的路径可能不同,需要根据实际情况进行修改。另外,在使用串口通讯时,需要保证Linux和stm32之间的串口参数一致,否则可能会导致通讯失败。
阅读全文
相关推荐
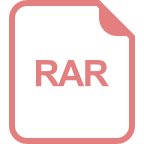
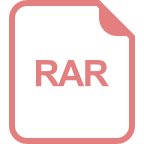
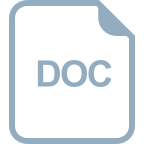
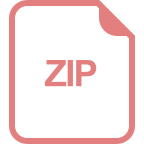
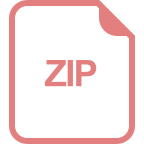
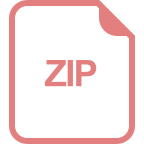
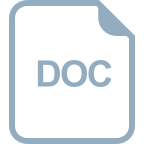
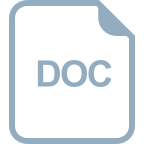
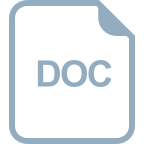
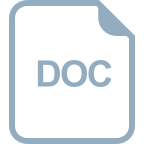
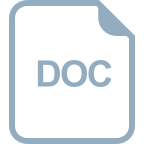
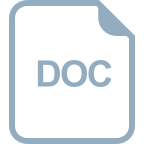
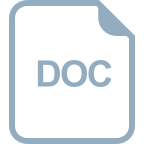
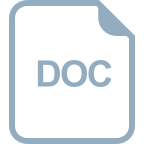