x = pyautogui.locateCenterOnScreen(im2, confidence=0.9)
时间: 2024-04-13 10:25:45 浏览: 169
你想问的是如何使用pyautogui库中的`locateCenterOnScreen`函数来定位图像在屏幕上的中心点,并设置置信度为0.9。
`locateCenterOnScreen`函数是pyautogui库中用于在屏幕上查找图像位置的函数。它接受一个图像路径或者一个PIL图像对象作为输入,并返回图像在屏幕上的中心点坐标。
在你的代码中,`im2`表示要查找的图像,而`confidence=0.9`表示置信度为0.9,即查找到的图像与给定图像匹配的最低置信度要求为0.9。
请确保已经安装了pyautogui库,并且已经导入了所需的模块和图像。运行这段代码后,变量`x`将会存储图像在屏幕上的中心点坐标。
相关问题
帮我优化我下面发送的代码,使图片识别过程中在屏幕上绘制出图片位置import tkinter as tk from tkinter import filedialog import pyautogui class Application(tk.Frame): def __init__(self, master=None): super().__init__(master) self.master = master self.pack() self.create_widgets() def create_widgets(self): self.select_button = tk.Button(self) self.select_button["text"] = "选择图片" self.select_button["command"] = self.select_image self.select_button.pack(side="top") self.result_label = tk.Label(self) self.result_label.pack(side="bottom") def select_image(self): # 弹出文件选择对话框选择要查找的图片 file_path = filedialog.askopenfilename(title="选择图片文件", filetypes=[("Image files", "*.png;*.jpg;*.jpeg")]) # 如果选择了图片则查找图片位置 if file_path: location = find_image_location(file_path) if location is not None: self.result_label["text"] = f"图片 {file_path} 的位置坐标为:{location}" else: self.result_label["text"] = f"图片 {file_path} 不存在于桌面" def find_image_location(image_path): # 加载图片并获取图片位置坐标 location = pyautogui.locateOnScreen(image_path,confidence=0.9) if location is not None: # 获取图片中心点的坐标 x, y = pyautogui.center(location) return (x, y) else: return None root = tk.Tk() app = Application(master=root) app.mainloop()
好的,你可以使用 pyautogui 库的 `screenshot()` 和 `drawRect()` 函数来实现在屏幕上绘制图片位置。
具体实现过程如下:
1. 在 find_image_location 函数中,在使用 pyautogui 库的 locateOnScreen 函数查找图片位置之前,先使用 pyautogui 库的 screenshot 函数获取当前屏幕的截图,并将其保存到变量 im 中。
2. 在使用 pyautogui 库的 locateOnScreen 函数查找图片位置之后,如果找到了图片,则使用 pyautogui 库的 drawRect 函数在屏幕上绘制出图片的位置矩形框。
3. 在使用 pyautogui 库的 drawRect 函数绘制矩形框时,需要传入图片位置的左上角坐标和右下角坐标,可以使用 pyautogui 库的 left、top、width 和 height 属性计算得到。
修改后的代码如下:
```
import tkinter as tk
from tkinter import filedialog
import pyautogui
class Application(tk.Frame):
def __init__(self, master=None):
super().__init__(master)
self.master = master
self.pack()
self.create_widgets()
def create_widgets(self):
self.select_button = tk.Button(self)
self.select_button["text"] = "选择图片"
self.select_button["command"] = self.select_image
self.select_button.pack(side="top")
self.result_label = tk.Label(self)
self.result_label.pack(side="bottom")
def select_image(self):
# 弹出文件选择对话框选择要查找的图片
file_path = filedialog.askopenfilename(title="选择图片文件", filetypes=[("Image files", "*.png;*.jpg;*.jpeg")])
# 如果选择了图片则查找图片位置
if file_path:
location = find_image_location(file_path)
if location is not None:
self.result_label["text"] = f"图片 {file_path} 的位置坐标为:{location}"
else:
self.result_label["text"] = f"图片 {file_path} 不存在于桌面"
def find_image_location(image_path):
# 获取当前屏幕截图
im = pyautogui.screenshot()
# 加载图片并获取图片位置坐标
location = pyautogui.locateOnScreen(image_path, confidence=0.9)
if location is not None:
# 获取图片位置的左上角和右下角坐标
left, top, width, height = location
right = left + width
bottom = top + height
# 在屏幕上绘制图片位置矩形框
pyautogui.drawRect(left, top, width, height)
# 获取图片中心点的坐标
x, y = pyautogui.center(location)
return (x, y)
else:
return None
root = tk.Tk()
app = Application(master=root)
app.mainloop()
```
这样,当程序找到图片位置时,会在屏幕上绘制出图片位置的矩形框,方便用户直观地了解图片位置。
阅读全文
相关推荐
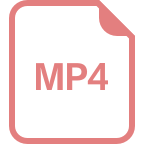
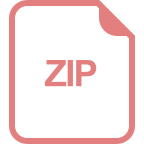
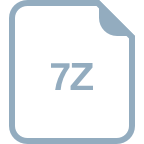
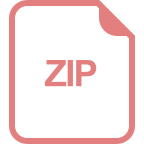
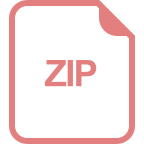
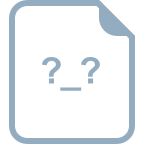
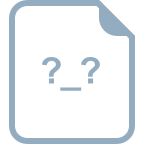
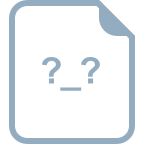
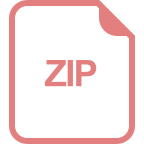
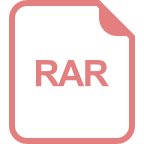