将字符串中指定的字母进行大小写转换,如果是大写则转换为小写,小写转换大写;
时间: 2023-05-25 11:02:50 浏览: 58
可以使用Python中的isupper()函数和islower()函数来判断字母是大写还是小写,然后使用upper()函数和lower()函数进行转换。
示例代码如下:
```python
def convert_str(s, letter):
res = ''
for c in s:
if c == letter:
if c.isupper():
c = c.lower()
else:
c = c.upper()
res += c
return res
s = 'Hello World!'
letter = 'o'
print(convert_str(s, letter))
```
输出结果为:
```
HellO WOrld!
```
在上面的示例代码中,convert_str()函数接受两个参数:字符串s和需要转换的字母letter。函数利用for循环遍历字符串中的每个字符,如果字符等于要转换的字母,就使用isupper()和islower()函数来判断字母大小写,然后使用upper()和lower()函数进行大小写转换。最后将转换好的字符添加到结果res字符串中,并返回结果。
相关问题
将字符串中指定的字母进行大小写转换,如果是大写则转换为小写,小写转换大写;
可以使用Python内置的字符串方法upper()和lower()来实现大小写转换。具体步骤如下:
1. 将字符串转换为列表,便于修改每个字符的大小写属性。
2. 使用循环遍历列表中的每个字符,如果是大写字符就使用lower()方法转换成小写字符,如果是小写字符就使用upper()方法转换成大写字符。
3. 将修改后的字符列表再转换为字符串。
示例代码如下:
```python
def change_case(s, c):
"""
将字符串中指定的字母进行大小写转换
:param s: 待转换的字符串
:param c: 指定转换的字母
:return: 转换后的字符串
"""
# 将字符串转换为列表
lst = list(s)
# 遍历列表,进行大小写转换
for i in range(len(lst)):
if lst[i] == c.upper():
lst[i] = c.lower()
elif lst[i] == c.lower():
lst[i] = c.upper()
# 将修改后的字符列表转换为字符串
return ''.join(lst)
```
示例测试:
```python
>>> change_case('The Quick Brown Fox', 'o')
'The Quick BrOwn FOx'
>>> change_case('The Quick Brown Fox', 'q')
'tHE QuiCK Brown Fox'
>>> change_case('The sun also rises.', 's')
'the Sun Also riSeS.'
>>> change_case('Life is like a box of chocolates.', 'x')
'Life is like a boX of chocolates.'
```
用c++实现将字符串中指定的字母进行大小写转换,如果是大写则转换为小写,小写转换大写;
#include <stdio.h>
#include <ctype.h>
void convert_case(char *str, char c) {
int i = 0;
while(str[i] != '\0') {
if(str[i] == c) { // 如果找到指定字母
if(isupper(str[i])) { // 如果是大写字母
str[i] = tolower(str[i]); // 转换为小写字母
} else { // 如果是小写字母
str[i] = toupper(str[i]); // 转换为大写字母
}
}
i++;
}
}
int main() {
char str[100];
char c;
printf("请输入一串字符串:");
gets(str);
printf("请输入要转换的字母:");
scanf("%c", &c);
convert_case(str, c);
printf("转换后的字符串为:%s\n", str);
return 0;
}
相关推荐
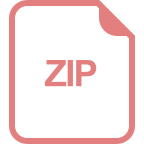












