elif self.level == 1: level_0_compressed = self.compress_level_0(x_level_0) level_0_resized = F.interpolate( level_0_compressed, scale_factor=2, mode='nearest') level_1_resized = x_level_1 level_2_resized = self.stride_level_2(x_level_2)
时间: 2023-09-11 16:06:59 浏览: 91
这段代码是在一个神经网络模型中,对于处理图像金字塔中第1层的情况进行定义。
具体来说,这段代码的作用如下:
1. 对于第1层,首先对输入的最底层图像`x_level_0`进行压缩操作,得到`level_0_compressed`。
2. 然后将`level_0_compressed`进行上采样操作,得到`level_0_resized`。上采样的方式为`F.interpolate`,采用最近邻插值的方式,将`level_0_compressed`的尺寸放大2倍。
3. 将第1层的中间层`x_level_1`直接赋值给`level_1_resized`。
4. 将第2层的下采样结果`level_2_resized`利用定义好的下采样层`self.stride_level_2`进行处理,得到下采样后的结果。
阅读全文
相关推荐
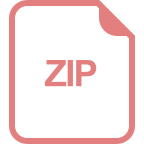
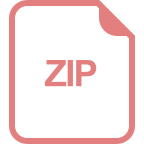
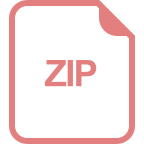
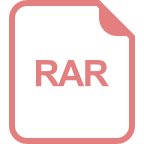
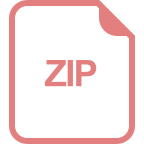
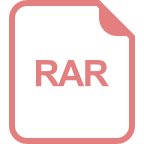
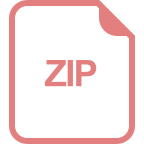
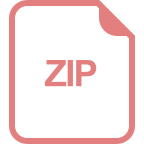
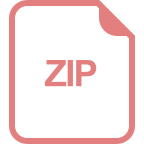
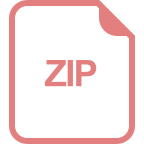
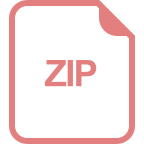