用linux C语言编写一个多线程访问sqlite内存数据库的例子。
时间: 2024-03-01 09:56:05 浏览: 151
下面是一个使用多线程访问SQLite内存数据库的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
#include <sqlite3.h>
#define THREAD_NUM 5
#define DB_NAME ":memory:"
sqlite3 *db;
void *thread_func(void *arg) {
int thread_id = *(int *)arg;
char sql[100];
printf("Thread %d: starting...\n", thread_id);
// 执行一些数据库操作
sprintf(sql, "INSERT INTO test VALUES (%d)", thread_id);
sqlite3_exec(db, sql, NULL, NULL, NULL);
printf("Thread %d: done!\n", thread_id);
pthread_exit(NULL);
}
int main() {
int i, ret;
pthread_t threads[THREAD_NUM];
int thread_ids[THREAD_NUM];
// 打开内存数据库
ret = sqlite3_open(DB_NAME, &db);
if (ret != SQLITE_OK) {
fprintf(stderr, "Failed to open database: %s\n", sqlite3_errmsg(db));
exit(1);
}
// 创建测试表
sqlite3_exec(db, "CREATE TABLE test (id INTEGER)", NULL, NULL, NULL);
// 创建多个线程来访问数据库
for (i = 0; i < THREAD_NUM; i++) {
thread_ids[i] = i;
ret = pthread_create(&threads[i], NULL, thread_func, &thread_ids[i]);
if (ret != 0) {
fprintf(stderr, "Failed to create thread %d\n", i);
exit(1);
}
}
// 等待所有线程完成
for (i = 0; i < THREAD_NUM; i++) {
pthread_join(threads[i], NULL);
}
// 查询测试表并输出结果
printf("Query results:\n");
sqlite3_exec(db, "SELECT * FROM test", [](void *data, int argc, char **argv, char **col_names) -> int {
for (int i = 0; i < argc; i++) {
printf("%s = %s\n", col_names[i], argv[i] ? argv[i] : "NULL");
}
printf("\n");
return 0;
}, NULL, NULL);
// 关闭数据库
sqlite3_close(db);
return 0;
}
```
该程序会创建一个内存数据库,并创建一个名为“test”的表,然后创建多个线程来访问数据库,在每个线程中插入一个整数值。最后,程序会查询“test”表并输出结果。
阅读全文
相关推荐
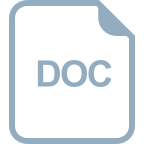
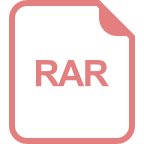
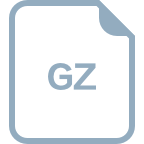
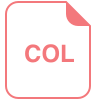
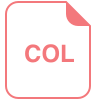
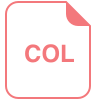
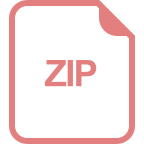
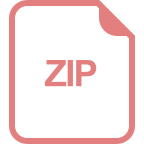
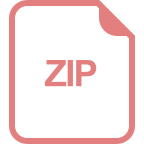
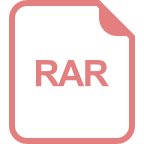
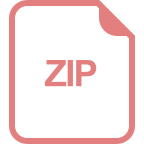