用于展示患者的就诊记录。每一条就诊记录需要展示就诊日期、医生姓名、诊断结果和处方信息。用户可以通过输入关键字进行搜索,搜索结果将实时展示在表格中。点击详情可以弹出一个弹窗组件,展示基本信息。 基本效果演示: VUE2实现
时间: 2024-05-04 14:17:55 浏览: 6
好的,这个需求可以用 Vue2 实现。首先,我们需要安装 Vue2:
```bash
npm install vue@2.6.14
```
接下来,我们可以用 Vue2 的单文件组件来实现这个需求。首先,我们创建一个 `Appointment.vue` 文件:
```html
<template>
<div>
<h2>就诊记录</h2>
<div class="search">
<input type="text" v-model="searchTerm" placeholder="请输入关键字">
<button @click="search">搜索</button>
</div>
<table>
<thead>
<tr>
<th>就诊日期</th>
<th>医生姓名</th>
<th>诊断结果</th>
<th>处方信息</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(appointment, index) in filteredAppointments" :key="appointment.id">
<td>{{ appointment.date }}</td>
<td>{{ appointment.doctor }}</td>
<td>{{ appointment.diagnosis }}</td>
<td>{{ appointment.prescription }}</td>
<td><button @click="showDetails(index)">详情</button></td>
</tr>
</tbody>
</table>
<div class="details" v-if="showDetailsPopup">
<div class="details-content">
<h3>{{ selectedAppointment.doctor }}</h3>
<p>{{ selectedAppointment.date }}</p>
<p>{{ selectedAppointment.diagnosis }}</p>
<p>{{ selectedAppointment.prescription }}</p>
<button @click="hideDetails">关闭</button>
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
appointments: [
{
id: 1,
date: '2021-08-01',
doctor: '张医生',
diagnosis: '感冒',
prescription: '999感冒灵',
},
{
id: 2,
date: '2021-08-03',
doctor: '李医生',
diagnosis: '发烧',
prescription: '退烧贴',
},
{
id: 3,
date: '2021-08-05',
doctor: '王医生',
diagnosis: '头痛',
prescription: '阿司匹林',
},
],
searchTerm: '',
showDetailsPopup: false,
selectedAppointment: null,
};
},
computed: {
filteredAppointments() {
if (this.searchTerm === '') {
return this.appointments;
}
return this.appointments.filter((appointment) => {
return (
appointment.date.includes(this.searchTerm) ||
appointment.doctor.includes(this.searchTerm) ||
appointment.diagnosis.includes(this.searchTerm) ||
appointment.prescription.includes(this.searchTerm)
);
});
},
},
methods: {
search() {
// do nothing
},
showDetails(index) {
this.selectedAppointment = this.filteredAppointments[index];
this.showDetailsPopup = true;
},
hideDetails() {
this.showDetailsPopup = false;
},
},
};
</script>
<style>
table {
border-collapse: collapse;
width: 100%;
}
th,
td {
border: 1px solid black;
padding: 8px;
text-align: left;
}
th {
background-color: #f2f2f2;
}
.search {
margin-bottom: 16px;
}
.details {
position: fixed;
top: 0;
left: 0;
width: 100%;
height: 100%;
background-color: rgba(0, 0, 0, 0.5);
display: flex;
justify-content: center;
align-items: center;
}
.details-content {
background-color: white;
padding: 16px;
border-radius: 8px;
box-shadow: 0 0 8px rgba(0, 0, 0, 0.3);
text-align: center;
}
.details-content h3 {
margin-top: 0;
}
.details-content p {
margin-bottom: 8px;
}
</style>
```
这个文件中包含一个 `Appointment` 组件,它包含一个表格用来展示就诊记录,一个搜索框和一个详情弹窗组件。我们在 `data` 中定义了一个 `appointments` 数组用来存储就诊记录,一个 `searchTerm` 用来存储用户输入的关键字,一个 `showDetailsPopup` 用来控制详情弹窗是否显示,一个 `selectedAppointment` 用来存储用户选择的就诊记录。
在 `computed` 中,我们定义了一个 `filteredAppointments` 计算属性,它根据用户输入的关键字来过滤就诊记录。在 `methods` 中,我们定义了一个 `search` 方法用来处理用户的搜索操作;一个 `showDetails` 方法用来展示详情弹窗,它将用户选择的就诊记录存储到 `selectedAppointment` 中,并将 `showDetailsPopup` 设置为 `true`;一个 `hideDetails` 方法用来关闭详情弹窗,它将 `showDetailsPopup` 设置为 `false`。
我们可以在父组件中使用 `Appointment` 组件来展示就诊记录:
```html
<template>
<div>
<appointment></appointment>
</div>
</template>
<script>
import Appointment from './Appointment.vue';
export default {
components: {
Appointment,
},
};
</script>
```
这样就完成了用 Vue2 实现展示患者的就诊记录的需求。
相关推荐
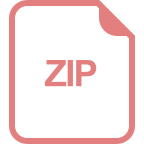
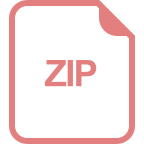
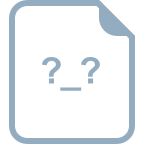














