winform设计复杂的倒计时功能
时间: 2023-07-30 21:04:38 浏览: 105
如果你需要设计一个复杂的倒计时功能,你可以使用 System.Threading 命名空间中的 Timer 类来实现。Timer 类和 Timer 控件不同,它是一个非 GUI 线程的计时器,可以在后台线程中进行计时。
下面是一个示例代码,实现了一个复杂的倒计时功能,可以设置倒计时时间、暂停、恢复和重置倒计时时间等功能:
```csharp
public partial class MainForm : Form
{
private int countdown = 0;
private bool isPaused = false;
private Timer timer;
public MainForm()
{
InitializeComponent();
}
private void buttonStart_Click(object sender, EventArgs e)
{
if (timer != null) // 如果 Timer 已经被创建,先停止它
{
timer.Dispose();
}
countdown = (int)numericUpDownTime.Value; // 获取用户设置的倒计时时间
timer = new Timer(1000); // 创建 Timer 实例,触发间隔为 1 秒
timer.Elapsed += Timer_Elapsed; // 绑定 Elapsed 事件处理函数
timer.Start(); // 启动 Timer
}
private void Timer_Elapsed(object sender, ElapsedEventArgs e)
{
if (isPaused) // 如果倒计时被暂停了,直接返回
{
return;
}
countdown--; // 减少倒计时时间
if (countdown < 0) // 如果倒计时时间已经为 0
{
timer.Stop(); // 停止 Timer
MessageBox.Show("时间到了!", "提示"); // 弹出提示框
return;
}
UpdateLabel(); // 更新 Label 控件的显示内容
}
private void buttonPause_Click(object sender, EventArgs e)
{
isPaused = true; // 标记倒计时被暂停了
}
private void buttonResume_Click(object sender, EventArgs e)
{
isPaused = false; // 标记倒计时已经恢复
}
private void buttonReset_Click(object sender, EventArgs e)
{
if (timer != null) // 如果 Timer 已经被创建,先停止它
{
timer.Dispose();
}
countdown = 0; // 重置倒计时时间为 0
UpdateLabel(); // 更新 Label 控件的显示内容
}
private void UpdateLabel()
{
if (labelTime.InvokeRequired) // 如果 Label 控件属于非 UI 线程
{
labelTime.Invoke(new Action(UpdateLabel)); // 使用 Invoke 方法将更新操作切换到 UI 线程
}
else
{
labelTime.Text = string.Format("倒计时:{0} 秒", countdown); // 更新 Label 控件的显示内容
}
}
}
```
在上面的代码中,我们使用了 Timer 类来实现一个复杂的倒计时功能。当用户点击开始按钮时,程序会创建一个 Timer 实例并且设置触发间隔为 1 秒。每次 Timer 触发 Elapsed 事件时,我们会检查是否被暂停,并且更新倒计时时间和 Label 控件的显示内容。当倒计时时间为 0 时,程序会停止 Timer 并且弹出提示框来提醒用户。在暂停、恢复和重置按钮的事件处理函数中,我们分别标记倒计时状态和重置倒计时时间,并且更新 Label 控件的显示内容。
需要注意的是,由于 Timer 类是一个非 GUI 线程的计时器,因此我们在更新 Label 控件的显示内容时需要使用 Invoke 方法将更新操作切换到 UI 线程。
阅读全文
相关推荐
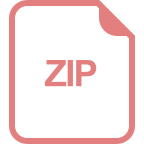
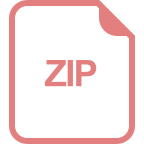
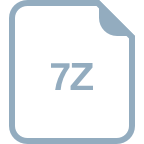
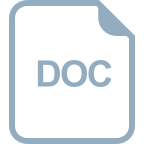
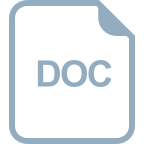
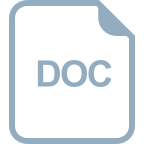
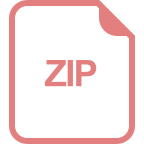
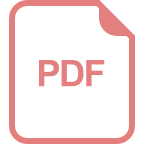