List<SchemaRowRule> userRowRuleList = schemaRowRuleList.stream() .filter(schemaRowRuleEo -> { List<String> userList = JSONArray.parseArray(schemaRowRuleEo.getRowPermissionUserList(), String.class); List<String> orgList = JSONArray.parseArray(schemaRowRuleEo.getRowPermissionOrgList(), String.class); return userList.contains(userUid) || orgList.contains(orgCode); }) .map(schemaRowRuleEo -> { SchemaRowRule schemaRowRule = SchemaRowRule.builder().build(); BeanUtils.copyProperties(schemaRowRuleEo, schemaRowRule); return schemaRowRule; }) .collect(Collectors.toList()); List<SchemaColumnRule> userColumnRuleList = schemaColumnRuleList.stream() .filter(rule -> { if (StringUtils.isNotEmpty(rule.getColumnPermissionUserList())) { List<String> userList = JSONArray.parseArray(rule.getColumnPermissionUserList(), String.class); return userList.contains(userUid); } else if (StringUtils.isNotEmpty(rule.getColumnPermissionOrgList())) { List<String> orgList = JSONArray.parseArray(rule.getColumnPermissionOrgList(), String.class); return orgList.contains(orgCode); } return false; }) .map(rule -> { SchemaColumnRule columnRule = new SchemaColumnRule(); BeanUtils.copyProperties(rule, columnRule); return columnRule; }) .collect(Collectors.toList());优化代码
时间: 2023-12-14 08:39:30 浏览: 175
可以考虑使用方法引用来简化Lambda表达式,同时避免多次解析JSON字符串,代码如下:
```
List<SchemaRowRule> userRowRuleList = schemaRowRuleList.stream()
.filter(schemaRowRuleEo -> {
List<String> userList = JSONArray.parseArray(schemaRowRuleEo.getRowPermissionUserList(), String.class);
List<String> orgList = JSONArray.parseArray(schemaRowRuleEo.getRowPermissionOrgList(), String.class);
return userList.contains(userUid) || orgList.contains(orgCode);
})
.map(schemaRowRuleEo -> {
SchemaRowRule schemaRowRule = new SchemaRowRule();
BeanUtils.copyProperties(schemaRowRuleEo, schemaRowRule);
return schemaRowRule;
})
.collect(Collectors.toList());
List<SchemaColumnRule> userColumnRuleList = schemaColumnRuleList.stream()
.filter(rule -> {
List<String> userList = Optional.ofNullable(rule.getColumnPermissionUserList())
.map(jsonArray -> JSONArray.parseArray(jsonArray, String.class))
.orElse(Collections.emptyList());
List<String> orgList = Optional.ofNullable(rule.getColumnPermissionOrgList())
.map(jsonArray -> JSONArray.parseArray(jsonArray, String.class))
.orElse(Collections.emptyList());
return userList.contains(userUid) || orgList.contains(orgCode);
})
.map(BeanUtils::instantiate)
.collect(Collectors.toList());
```
其中,使用了`Optional`来避免`null`值的判断和处理,使用`BeanUtils::instantiate`方法引用来创建对象并复制属性。
阅读全文
相关推荐
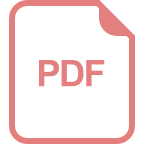
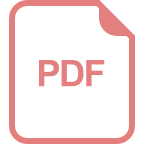
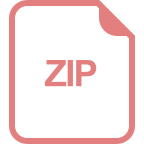













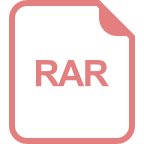
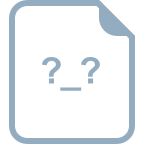
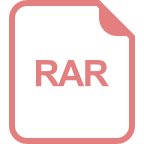