用c++写一个识别到图片自动删除的脚本代码
时间: 2024-03-11 11:49:27 浏览: 140
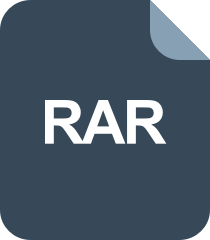
通过 代码 识别 图片
以下是使用C++编写的一个可以识别并删除指定图片的代码示例:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
#include <filesystem>
using namespace std;
using namespace cv;
namespace fs = std::filesystem;
void removeImages(const string& folderPath, const string& imagePath) {
// 加载待删除的图片
Mat imgToRemove = imread(imagePath);
// 遍历文件夹中的所有图片
for (const auto& entry : fs::directory_iterator(folderPath)) {
if (entry.path().extension() == ".jpg" || entry.path().extension() == ".png") {
// 加载当前图片
Mat img = imread(entry.path().string());
// 如果两张图片相同,则删除当前图片
if (countNonZero(img != imgToRemove) == 0) {
remove(entry.path().string().c_str());
}
}
}
}
int main() {
// 文件夹路径
string folderPath = "/path/to/folder";
// 待删除的图片路径
string imagePath = "/path/to/image";
// 删除图片
removeImages(folderPath, imagePath);
return 0;
}
```
在代码中,您需要指定文件夹路径和待删除的图片路径。然后,代码将遍历文件夹中的所有图片,并使用OpenCV库加载它们。如果当前图片与待删除的图片相同,则它将被删除。
请注意,该代码只能删除完全相同的图片。如果两张图片有微小的差异,则它们不会被检测和删除。
阅读全文
相关推荐
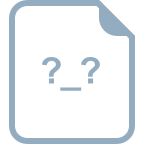
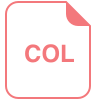
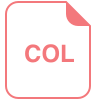
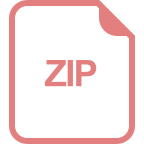
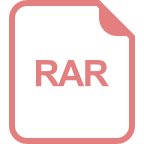
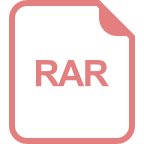
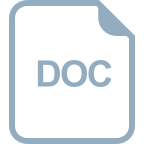
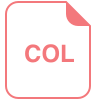
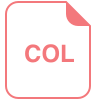
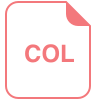
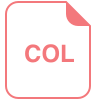
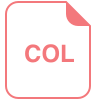
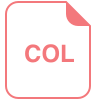
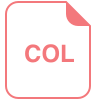
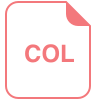
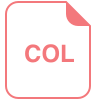