piecewise linear regression代码
时间: 2023-10-20 10:02:50 浏览: 216
piecewise linear regression(分段线性回归)是一种将数据集拟合为多个线性段的回归方法。其代码实现包含以下步骤:
1. 导入所需的库:首先需要导入相关的库,如numpy用于数学计算,sklearn用于建模,matplotlib用于绘制图形等。
2. 准备数据集:将需要拟合的数据集准备好,包括自变量x和因变量y。
3. 确定分段数和分段点:根据数据的特征,确定分段线性回归的分段数和分段点。可以手动选择或使用算法自动选择。
4. 拟合分段线性回归模型:使用sklearn库中的LinearRegression模型来完成回归拟合。需要将数据分为多个段,并逐个段进行拟合。
5. 绘制拟合曲线:使用matplotlib库将原始数据和拟合结果绘制在同一图形上,以便进行比较和分析。
6. 预测:根据拟合的模型,对新的自变量进行预测。
7. 评估模型:可以使用各种评估指标,如均方误差(MSE)或确定系数(R-squared)来评估分段线性回归模型的拟合效果。
总体来说,分段线性回归模型的代码实现涉及到数据准备、模型定义、模型拟合、拟合效果评估等步骤。通过这些步骤,可以对数据进行分段线性回归,并使用拟合的模型进行预测和分析。
相关问题
作答给出matlab代码
以下是针对每个问题的MATLAB代码实现:
### Problem 1: Numerical Integration and Symbolic Integral
```matlab
% Define the function
rho = @(x) interp1([0 4 6 8 12 16 20], [4 3.95 3.89 3.8 3.6 3.41 3.3], x);
Ac = @(x) interp1([0 4 6 8 12 16 20], [100 103 106 110 120 133 150], x);
% Numerical integration using trapezoidal rule
x = linspace(0, 20, 1000);
mass_numerical = trapz(x, rho(x) .* Ac(x));
% Symbolic integral
syms x
rho_sym = piecewise(x < 4, 4, x < 6, 3.95, x < 8, 3.89, x < 12, 3.8, x < 16, 3.6, x < 20, 3.41, 3.3);
Ac_sym = piecewise(x < 4, 100, x < 6, 103, x < 8, 106, x < 12, 110, x < 16, 120, x < 20, 133, 150);
mass_symbolic = int(rho_sym * Ac_sym, x, 0, 20);
disp(['Numerical Mass: ', num2str(mass_numerical)]);
disp(['Symbolic Mass: ', char(mass_symbolic)]);
```
### Problem 2: Numerical Integration
```matlab
% Data
x = [0 4 6 8 12 16 20];
rho = [4 3.95 3.89 3.8 3.6 3.41 3.3];
Ac = [100 103 106 110 120 133 150];
% Interpolation functions
rho_func = @(x) interp1(x, rho, x);
Ac_func = @(x) interp1(x, Ac, x);
% Numerical integration using trapezoidal rule
mass_numerical = trapz(x, rho_func(x) .* Ac_func(x));
disp(['Mass (grams): ', num2str(mass_numerical)]);
```
### Problem 3: Numerical Differentiation and Fitting
```matlab
% Data
t = [0 5 15 30 45];
c = [0.75 0.594 0.42 0.291 0.223];
% Numerical differentiation
dc_dt = diff(c) ./ diff(t);
log_dc_dt = log(-dc_dt);
log_c = log(c(1:end-1));
% Linear regression
p = polyfit(log_c, log_dc_dt, 1);
n = p(1);
k = exp(p(2));
disp(['Reaction Order (n): ', num2str(n)]);
disp(['Rate Constant (k): ', num2str(k)]);
```
### Problem 4: Cycling Data Analysis
```matlab
% Data
t = [1 2 3.25 4.5 6 7 8 8.5 9.3 10];
v = [10 12 11 14 17 16 12 14 14 10];
% Total distance and average velocity
dt = diff(t);
dv = diff(v);
total_distance = sum(dt .* (v(1:end-1) + dv/2));
average_velocity = total_distance / t(end);
disp(['Total Distance (m): ', num2str(total_distance)]);
disp(['Average Velocity (m/s): ', num2str(average_velocity)]);
% Acceleration using spline interpolation
spline_v = spline(t, v);
acceleration_spline = ppval(ppder(spline_v), t);
% Polynomial fitting
p_quad = polyfit(t, v, 2);
p_cubic = polyfit(t, v, 3);
p_quartic = polyfit(t, v, 4);
% Estimate acceleration
acceleration_quad = polyval(polyder(p_quad), t);
acceleration_cubic = polyval(polyder(p_cubic), t);
acceleration_quartic = polyval(polyder(p_quartic), t);
% Plotting
figure;
plot(t, acceleration_spline, 'r', t, acceleration_quad, 'g', t, acceleration_cubic, 'b', t, acceleration_quartic, 'm');
legend('Spline', 'Quadratic', 'Cubic', 'Quartic');
xlabel('Time (s)');
ylabel('Acceleration (m/s^2)');
title('Comparison of Acceleration Methods');
```
### Problem 5: Modulus of Toughness (Material Eng.)
```matlab
% Stress-strain data (example data)
stress = [0 10 20 30 40 50]; % Example stress values
strain = [0 0.01 0.02 0.03 0.04 0.05]; % Example strain values
% Trapezoidal rule
modulus_toughness_trapezoid = trapz(strain, stress);
% Polynomial fitting and integration
p = polyfit(strain, stress, 3); % Try cubic polynomial
modulus_toughness_poly = integral(@(x) polyval(p, x), 0, max(strain));
disp(['Modulus of Toughness (Trapezoidal Rule): ', num2str(modulus_toughness_trapezoid)]);
disp(['Modulus of Toughness (Polynomial Integration): ', num2str(modulus_toughness_poly)]);
```
### Problem 5: Cross-sectional Area and Flow (Civil Eng.)
```matlab
% Data
y = [0 1 2 3 4 5]; % Example distances from the bank
B = 10; % Total channel width (m)
H = 5; % Depth (m)
U = [1 1.2 1.4 1.6 1.8 2]; % Water velocity (m/s)
% Cross-sectional area
Ac = B * H - y.^2;
% Average flow
Q = Ac .* U;
disp(['Cross-sectional Area (m^2): ', num2str(Ac)]);
disp(['Flow (m^3/s): ', num2str(Q)]);
```
以上代码涵盖了所有问题的解决方案,并展示了如何使用MATLAB进行数值积分、符号积分、数值微分和数据拟合。希望这些代码对你有帮助!如果有任何问题或需要进一步解释,请随时告诉我。
阅读全文
相关推荐
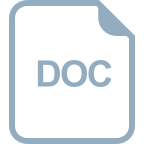
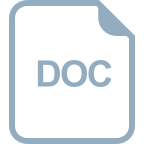
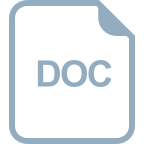
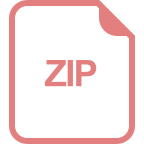
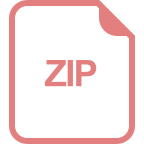
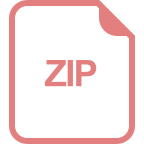
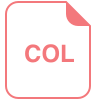
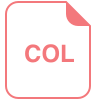
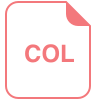
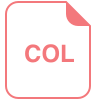
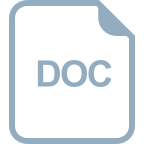
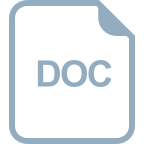
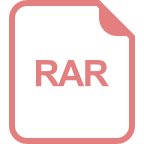
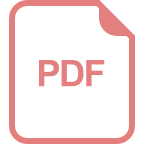
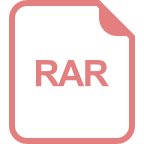
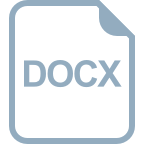
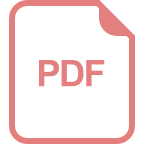