springboot实现富文本html转word(近乎百分之百还原)
时间: 2023-05-08 16:58:52 浏览: 1408
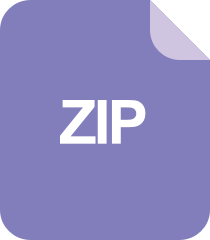
SpringBoot+thymeleaf
Spring Boot可以通过使用Apache POI和Thymeleaf模板引擎来实现富文本HTML转Word,最终达到近乎百分之百的还原效果。
首先,需要在pom.xml文件中添加Apache POI和Thymeleaf的依赖:
```
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>4.1.1</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>4.1.1</version>
</dependency>
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>ooxml-schemas</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf</artifactId>
<version>3.0.11.RELEASE</version>
</dependency>
<dependency>
<groupId>org.thymeleaf</groupId>
<artifactId>thymeleaf-spring5</artifactId>
<version>3.0.11.RELEASE</version>
</dependency>
```
接着,需要编写一个自定义Thymeleaf模板引擎,用于将HTML生成Word格式的文件。
```
@Configuration
public class ThymeleafConfig {
@Bean
public TemplateEngine templateEngine() {
SpringTemplateEngine engine = new SpringTemplateEngine();
engine.setTemplateResolver(templateResolver());
engine.addDialect(new LayoutDialect());
return engine;
}
private ITemplateResolver templateResolver() {
ServletContextTemplateResolver resolver = new ServletContextTemplateResolver();
resolver.setPrefix("/WEB-INF/templates/");
resolver.setTemplateMode("HTML5");
resolver.setSuffix(".html");
resolver.setCharacterEncoding("UTF-8");
resolver.setOrder(0);
resolver.setCacheable(false);
return resolver;
}
@Bean
public ViewResolver viewResolver() {
ThymeleafViewResolver viewResolver = new ThymeleafViewResolver();
viewResolver.setTemplateEngine(templateEngine());
viewResolver.setCharacterEncoding("UTF-8");
viewResolver.setOrder(1);
return viewResolver;
}
}
```
然后,就可以编写一个Controller来处理HTML转Word的请求。
```
@RequestMapping(value = "/htmlToWord", method = RequestMethod.GET)
public void htmlToWord(HttpServletResponse response) {
response.setContentType("application/msword");
response.setHeader("Content-Disposition", "attachment; filename=document.docx");
OutputStream out = null;
try {
out = response.getOutputStream();
Context ctx = new Context();
//将HTML模板中的数据填充到模板中
String html = templateEngine.process("document", ctx);
//使用Apache POI将HTML转换为Word
XWPFDocument document = new XWPFDocument();
String strArr[] = html.split("\n");
for (String str : strArr) {
if (str.contains("<p>")) {
String s = str.replaceAll("<[^>]*>", "");
XWPFParagraph paragraph = document.createParagraph();
XWPFRun run = paragraph.createRun();
run.setText(s);
}
}
document.write(out);
out.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (out != null) {
try {
out.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
```
最后,编写HTML模板文件,可以使用一些CSS样式来美化页面。
```
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Document</title>
<style type="text/css">
body {
font-family: "微软雅黑";
font-size: 16px;
}
h1 {
font-size: 34px;
font-weight: bold;
text-align: center;
}
</style>
</head>
<body>
<h1>这是一个HTML转Word的示例</h1>
<p>富文本HTML转Word的效果如何呢?</p>
<p>多种<b>样式</b>以及图片的展示:</p>
<div><img src="https://example.com/image.jpg" alt="" style="width: 100%;"></div>
<p>除了图片,还可以展示表格:</p>
<table>
<tr>
<th>姓名</th>
<th>年龄</th>
<th>性别</th>
</tr>
<tr>
<td>张三</td>
<td>20</td>
<td>男</td>
</tr>
<tr>
<td>李四</td>
<td>30</td>
<td>女</td>
</tr>
</table>
</body>
</html>
```
通过以上步骤,Spring Boot项目就可以实现富文本HTML转Word的功能了。
阅读全文
相关推荐
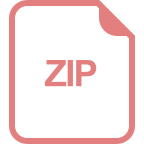
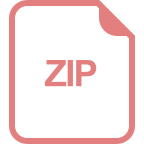
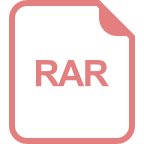
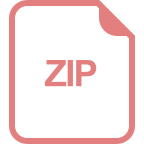
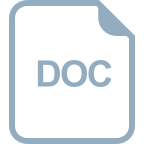
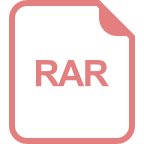
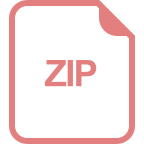
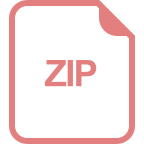
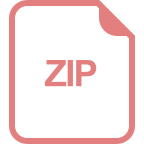
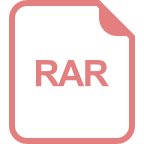
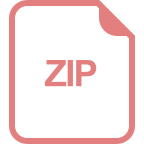
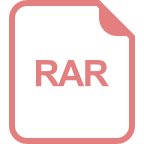
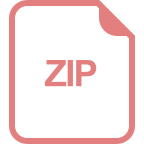