vcpkg 使用无锁队列
时间: 2023-07-11 20:23:18 浏览: 127
vcpkg 中使用的无锁队列是一个基于 CAS (Compare and Swap) 操作的实现,它的实现位于 vcpkg 的 `include/vcpkg/fwdlock.h` 头文件中。
无锁队列的实现使用了 C++11 中的 `std::atomic` 类型,它支持原子操作,并且可以避免多线程访问时的竞争问题。在无锁队列中,每个节点包含一个数据项和一个指向下一个节点的指针,节点的插入和删除操作都是通过原子 CAS 操作来实现的。
无锁队列的代码示例:
```c++
template <typename T>
class fwdlock {
public:
fwdlock() : head_(nullptr), tail_(nullptr) {}
void push(T data) {
node* n = new node(std::move(data));
node* t = tail_.load(std::memory_order_relaxed);
do {
n->next_ = t;
} while (!tail_.compare_exchange_weak(t, n, std::memory_order_release, std::memory_order_relaxed));
}
bool try_pop(T& data) {
node* h = head_.load(std::memory_order_relaxed);
node* t = tail_.load(std::memory_order_acquire);
if (h == t) {
return false;
}
node* next = h->next_;
data = std::move(next->data_);
head_.store(next, std::memory_order_release);
delete h;
return true;
}
private:
struct node {
T data_;
node* next_;
node(T data) : data_(std::move(data)), next_(nullptr) {}
};
std::atomic<node*> head_;
std::atomic<node*> tail_;
};
```
在上述代码中,`fwdlock` 类实现了无锁队列的 push 和 try_pop 操作,其中 push 操作使用了 `compare_exchange_weak` 原子操作,try_pop 操作使用了 `load` 和 `store` 原子操作。使用无锁队列可以有效减少多线程并发访问时的竞争和锁等待,从而提高代码的性能和可伸缩性。
阅读全文
相关推荐
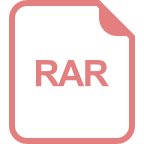
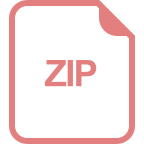



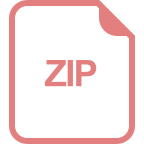
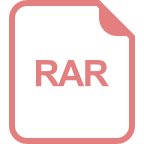









