c语言 创建链表函数
时间: 2024-04-05 22:27:41 浏览: 152
在C语言中,创建链表的函数通常包括以下几个步骤:
1. 定义链表节点的结构体:首先需要定义一个结构体,用来表示链表的节点。结构体中通常包含一个数据域和一个指向下一个节点的指针域。
```c
struct Node {
int data;
struct Node* next;
};
```
2. 创建链表头节点:链表的头节点是链表的起始位置,它不存储任何数据,只是用来指向第一个实际节点。
```c
struct Node* createLinkedList() {
struct Node* head = (struct Node*)malloc(sizeof(struct Node));
head->next = NULL;
return head;
}
```
3. 添加节点到链表:可以编写一个函数来添加新节点到链表中。该函数需要接收链表头节点和要添加的数据作为参数。
```c
void addNode(struct Node* head, int data) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->data = data;
newNode->next = NULL;
struct Node* current = head;
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
```
4. 打印链表:可以编写一个函数来遍历链表并打印每个节点的数据。
```c
void printLinkedList(struct Node* head) {
struct Node* current = head->next;
while (current != NULL) {
printf("%d ", current->data);
current = current->next;
}
printf("\n");
}
```
使用以上函数,你可以创建一个链表并添加节点,然后打印链表的内容。
阅读全文
相关推荐
















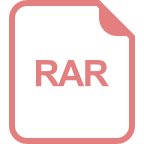