vhdl std_logic_vector 加法
时间: 2023-10-13 14:05:48 浏览: 131
std_logic_vector加法在VHDL中是通过使用`ieee.numeric_std`库中的`unsigned`数据类型来完成的。可以使用`&`运算符将两个`std_logic_vector`相加。下面是一个示例的代码片段:
```
library ieee;
use ieee.std_logic_1164.all;
use ieee.numeric_std.all;
entity adder is
port (
a, b: in std_logic_vector(3 downto 0);
sum: out std_logic_vector(3 downto 0)
);
end entity adder;
architecture rtl of adder is
begin
sum <= std_logic_vector(unsigned(a) + unsigned(b));
end architecture rtl;
```
在上面的代码中,`std_logic_vector`类型的信号`a`和`b`被转换为`unsigned`类型,并使用`+`运算符进行相加。然后,通过使用`std_logic_vector`函数将结果转换回`std_logic_vector`类型,并赋值给`sum`信号。
相关问题
vhdl std_logic_vector加法运算的例子
下面是一个简单的vhdl std_logic_vector加法运算的例子:
```
library IEEE;
use IEEE.STD_LOGIC_1164.ALL;
use IEEE.NUMERIC_STD.ALL;
entity adder is
Port ( a : in std_logic_vector(3 downto 0);
b : in std_logic_vector(3 downto 0);
sum : out std_logic_vector(4 downto 0);
carry : out std_logic);
end adder;
architecture Behavioral of adder is
begin
process(a,b)
variable temp_sum : unsigned(3 downto 0);
variable temp_carry : std_logic;
begin
temp_sum := (unsigned(a) + unsigned(b));
if (temp_sum > 15) then
temp_carry := '1';
else
temp_carry := '0';
end if;
sum <= std_logic_vector(temp_sum);
carry <= temp_carry;
end process;
end Behavioral;
```
在这个例子中,我们设计了一个名为“adder”的实体,它有两个4位的输入端口a和b,一个5位的输出端口sum和一个输出端口carry。在architecture中,我们使用了一个process来执行加法运算,并将结果赋值给输出端口。在process中,我们首先声明了两个变量temp_sum和temp_carry,temp_sum是一个unsigned类型,用来存储a和b的和,temp_carry是一个标准逻辑类型,用来表示进位。然后我们使用了加法运算符将a和b转化为unsigned类型,执行加法运算后将结果存储在temp_sum中。接下来,我们通过比较temp_sum的值是否大于15来判断是否有进位,如果有,则将temp_carry赋值为'1',否则赋值为'0'。最后我们将temp_sum转化为std_logic_vector类型并赋值给输出端口sum,将temp_carry赋值给输出端口carry。
以上是一个简单的vhdl std_logic_vector加法运算的例子,通过类似的方法,我们可以实现更复杂的运算。
vhdl std_logic_vector的简单加法赋值
下面是使用VHDL实现std_logic_vector简单加法赋值的一个例子:
```vhdl
library ieee;
use ieee.std_logic_1164.all;
entity adder is
port (a, b : in std_logic_vector(3 downto 0);
c : out std_logic_vector(4 downto 0));
end entity;
architecture behavioral of adder is
begin
c <= ('0' & a) + ('0' & b); -- 加法运算,注意位对齐
end architecture;
```
在上面的代码中,使用了位连接运算符“&”,将一个0位添加到std_logic_vector的最高位,以保证位对齐。然后,将两个std_logic_vector相加,将结果赋值给输出std_logic_vector。
值得注意的是,在使用std_logic_vector进行加法运算时,“0”会自动转换为逻辑0,而“1”会自动转换为逻辑1。因此,在进行加法运算时,不需要显式地进行数字转换。
相关推荐
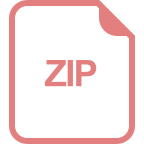
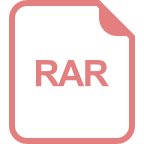












