C# 如何对浮点数进行 CORDIC
时间: 2024-11-24 18:44:04 浏览: 7
CORDIC(Coordinate Rotation Digital Computer)算法是一种用于计算三角函数、双曲线函数、指数和对数等数学函数的迭代算法。在C#中,我们可以使用CORDIC算法来处理浮点数运算。
以下是一个简单的C#示例,演示如何使用CORDIC算法来计算给定角度的正弦值:
```csharp
using System;
class Program
{
static void Main()
{
double angle = 45.0; // 角度
double result = CordicSin(angle);
Console.WriteLine("Sin(45) = " + result);
}
static double CordicSin(double angle)
{
double x = 1.0;
double y = 0.0;
double z = angle * Math.PI / 180.0; // 将角度转换为弧度
double currentAngle = Math.Atan(1.0); // 初始角度为45度
for (int i = 0; i < 20; i++) // 迭代次数
{
if (z > 0)
{
double newX = x - y * Math.Tan(currentAngle);
y = x * Math.Tan(currentAngle) + y;
x = newX;
z -= currentAngle;
}
else
{
double newX = x + y * Math.Tan(currentAngle);
y = x * Math.Tan(currentAngle) - y;
x = newX;
z += currentAngle;
}
currentAngle /= 2.0; // 更新当前角度
}
return y; // 返回正弦值
}
}
```
在这个例子中,我们定义了一个`CordicSin`方法来计算给定角度的正弦值。我们首先将角度从度转换为弧度,然后初始化变量`x`和`y`。通过多次迭代,我们逐渐逼近目标角度,并更新`x`和`y`的值。最终,`y`的值就是所求的正弦值。
请注意,这个示例仅用于演示如何在C#中使用CORDIC算法进行基本的浮点数运算。在实际应用中,可能需要更复杂的实现和优化以达到更高的精度和性能。
阅读全文
相关推荐
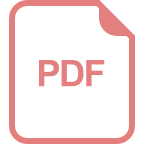
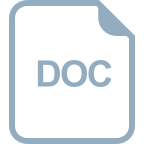
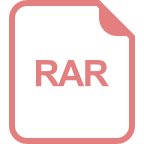
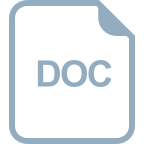


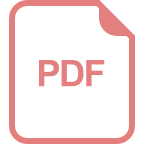
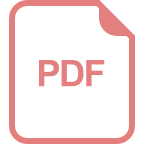
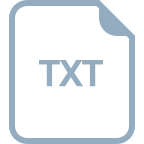
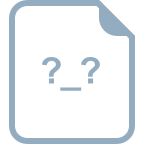
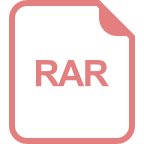
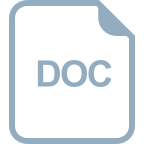
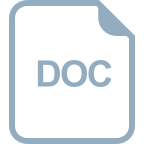
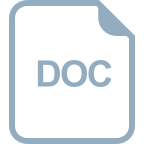
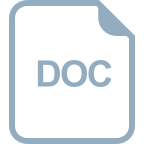

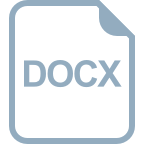