编写程序输入一批学生数据链式存放,然后输出这批学生中的分数最高的学生信息 C语言
时间: 2024-09-27 07:13:31 浏览: 77
在C语言中,我们可以使用链表结构来存储学生的数据,每个节点包含学生的信息(如姓名和分数)。为了找到分数最高的学生,我们需要遍历链表并保持当前最高分及其对应的节点。以下是实现这个功能的一个简化的示例:
```c
#include <stdio.h>
#include <stdlib.h>
typedef struct Student {
char name[50];
int score;
struct Student* next; // 指向下一个学生的指针
} Student;
// 初始化链表
void initList(Student** head) {
*head = (Student*)malloc(sizeof(Student));
if (*head == NULL) {
printf("Memory allocation failed.\n");
exit(1);
}
(*head)->score = -1; // 初始最高分为-1
(*head)->next = NULL;
}
// 比较并更新最高分及对应的学生节点
void updateMaxScore(Student* current, Student** maxHead) {
if (current->score > (*maxHead)->score) {
Student* temp = *maxHead;
*maxHead = current;
free(temp); // 释放旧的最高分节点的内存
}
}
// 添加学生到链表
void addStudent(char* name, int score, Student** head) {
Student* newNode = (Student*)malloc(sizeof(Student));
if (newNode == NULL) {
printf("Memory allocation failed.\n");
exit(1);
}
strcpy(newNode->name, name);
newNode->score = score;
newNode->next = (*head);
*head = newNode;
}
// 遍历链表并输出最高分学生信息
void printHighestScore(Student* head) {
Student* maxHead = head;
while (maxHead != NULL) {
updateMaxScore(maxHead, &maxHead);
maxHead = maxHead->next;
}
if (maxHead == NULL) { // 如果链表为空
printf("No students found.\n");
} else {
printf("Highest score student: Name - %s, Score - %d\n", maxHead->name, maxHead->score);
}
}
int main() {
Student* head = NULL;
initList(&head);
// 添加学生数据,例如:
addStudent("Alice", 90, &head);
addStudent("Bob", 85, &head);
addStudent("Charlie", 95, &head);
printHighestScore(head);
return 0;
}
```
在这个程序中,我们首先初始化一个空链表,并定义了添加学生、比较更新最高分以及打印最高分学生的方法。在`main`函数中,你可以根据实际需求添加更多的学生。
阅读全文
相关推荐



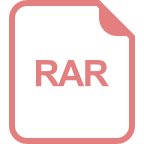


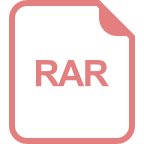

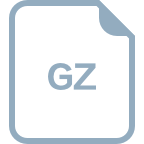

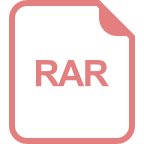


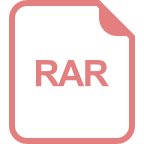
