easypoi中上传文件方式excel导入
时间: 2023-10-01 08:03:37 浏览: 97
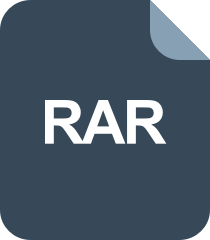
springboot整合easypoi实现文件导入导出OSS文件上传和下载。OSS图片导出
1. 引入easypoi和上传文件的依赖:
```xml
<!-- 引入easypoi -->
<dependency>
<groupId>cn.afterturn</groupId>
<artifactId>easypoi-base</artifactId>
<version>4.2.0</version>
</dependency>
<!-- 文件上传依赖 -->
<dependency>
<groupId>commons-fileupload</groupId>
<artifactId>commons-fileupload</artifactId>
<version>1.4</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-io</artifactId>
<version>1.3.2</version>
</dependency>
```
2. 创建一个Excel导入的POJO类,例如:
```java
import cn.afterturn.easypoi.excel.annotation.Excel;
import lombok.Data;
@Data
public class UserExcelModel {
@Excel(name = "姓名")
private String name;
@Excel(name = "年龄")
private Integer age;
@Excel(name = "性别")
private String gender;
}
```
3. 创建Controller处理上传并导入Excel的请求:
```java
import cn.afterturn.easypoi.excel.ExcelImportUtil;
import cn.afterturn.easypoi.excel.entity.ImportParams;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.io.FileUtils;
import org.apache.commons.io.IOUtils;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.multipart.MultipartFile;
import javax.servlet.http.HttpServletResponse;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
@Slf4j
@Controller
@RequestMapping("/excel")
public class ExcelController {
@PostMapping("/import")
public void importExcel(@RequestParam("file") MultipartFile file,
HttpServletResponse response) throws IOException {
// 将MultipartFile转换为File
File tempFile = File.createTempFile("temp", null);
FileUtils.copyInputStreamToFile(file.getInputStream(), tempFile);
// 导入Excel
ImportParams importParams = new ImportParams();
List<UserExcelModel> userList = ExcelImportUtil.importExcel(
tempFile, UserExcelModel.class, importParams);
// 做一些业务处理,例如将数据保存到数据库
// 返回结果给前端
response.setContentType("application/json;charset=UTF-8");
response.getWriter().write("导入成功!");
}
}
```
4. 在前端页面中添加一个文件上传表单,并将文件上传到Controller中:
```html
<form action="/excel/import" method="post" enctype="multipart/form-data">
<input type="file" name="file">
<button type="submit">上传并导入</button>
</form>
```
阅读全文
相关推荐
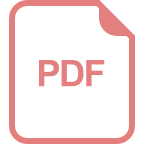
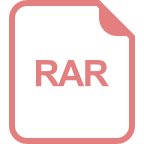















