html5制作音乐播放界面
时间: 2023-08-29 13:06:03 浏览: 76
HTML5 提供了 `<audio>` 元素,可以用来播放音频。下面是一个简单的音乐播放器界面的 HTML 代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>音乐播放器</title>
<meta charset="UTF-8">
<style>
.container {
display: flex;
flex-direction: column;
align-items: center;
margin-top: 50px;
}
.cover {
width: 300px;
height: 300px;
background-color: #eee;
border-radius: 50%;
display: flex;
justify-content: center;
align-items: center;
margin-bottom: 20px;
}
.cover img {
max-width: 100%;
max-height: 100%;
border-radius: 50%;
}
.controls {
display: flex;
align-items: center;
margin-bottom: 20px;
}
.controls button {
margin-right: 10px;
border: none;
background-color: #ccc;
padding: 10px;
border-radius: 5px;
cursor: pointer;
}
.controls button:hover {
background-color: #999;
}
.progress {
height: 5px;
background-color: #ccc;
width: 100%;
margin-bottom: 20px;
position: relative;
}
.progress-bar {
position: absolute;
top: 0;
left: 0;
height: 100%;
background-color: #333;
width: 0;
}
.time {
display: flex;
justify-content: space-between;
align-items: center;
width: 100%;
font-size: 12px;
margin-bottom: 20px;
}
.time span {
color: #999;
}
</style>
</head>
<body>
<div class="container">
<div class="cover">
<img src="封面图片地址" alt="封面">
</div>
<div class="controls">
<button id="prev">上一首</button>
<button id="play">播放</button>
<button id="next">下一首</button>
</div>
<div class="progress">
<div class="progress-bar"></div>
</div>
<div class="time">
<span id="current-time">00:00</span>
<span id="duration">00:00</span>
</div>
</div>
<script>
var audio = new Audio("音乐地址");
var playBtn = document.getElementById("play");
var prevBtn = document.getElementById("prev");
var nextBtn = document.getElementById("next");
var progressBar = document.querySelector(".progress-bar");
var currentTime = document.getElementById("current-time");
var duration = document.getElementById("duration");
playBtn.addEventListener("click", function() {
if (audio.paused) {
audio.play();
playBtn.textContent = "暂停";
} else {
audio.pause();
playBtn.textContent = "播放";
}
});
audio.addEventListener("timeupdate", function() {
var progress = audio.currentTime / audio.duration * 100;
progressBar.style.width = progress + "%";
var minutes = Math.floor(audio.currentTime / 60);
var seconds = Math.floor(audio.currentTime % 60);
if (minutes < 10) {
minutes = "0" + minutes;
}
if (seconds < 10) {
seconds = "0" + seconds;
}
currentTime.textContent = minutes + ":" + seconds;
});
audio.addEventListener("loadedmetadata", function() {
var minutes = Math.floor(audio.duration / 60);
var seconds = Math.floor(audio.duration % 60);
if (minutes < 10) {
minutes = "0" + minutes;
}
if (seconds < 10) {
seconds = "0" + seconds;
}
duration.textContent = minutes + ":" + seconds;
});
prevBtn.addEventListener("click", function() {
// 切换到上一首
});
nextBtn.addEventListener("click", function() {
// 切换到下一首
});
</script>
</body>
</html>
```
需要注意的是,这段代码只提供了播放、暂停、进度条和时间显示的基本功能,如果需要添加其他功能(例如切换歌曲),需要自行编写 JavaScript 代码实现。
阅读全文
相关推荐









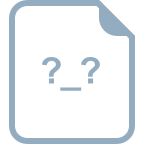
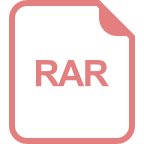
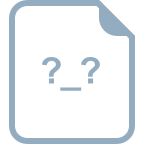
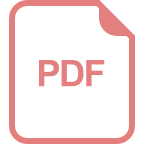
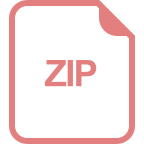
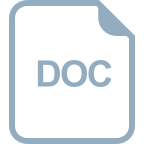