使用反射 获取到继承类的属性与方法 并调用,以及实现接口的方法 代码请提供详细点
时间: 2024-02-18 07:03:17 浏览: 64
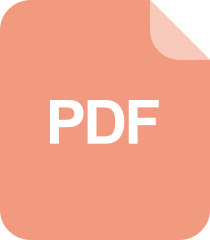
Java 采用反射获取class属性值的实现代码
好的,以下是一个示例代码,可以用来演示如何使用反射获取继承类的属性和方法,并调用这些属性和方法,以及实现接口的方法:
```python
class Animal:
def __init__(self, name, sound):
self.name = name
self.sound = sound
def speak(self):
print(f"{self.name} says {self.sound}")
class Dog(Animal):
def __init__(self, name):
super().__init__(name, "woof")
def run(self):
print(f"{self.name} is running")
class Cat(Animal):
def __init__(self, name):
super().__init__(name, "meow")
def scratch(self):
print(f"{self.name} is scratching")
class AnimalInterface:
def speak(self):
pass
def run(self):
pass
def scratch(self):
pass
class AnimalProxy:
def __init__(self, animal):
self.animal = animal
def __getattr__(self, attr):
if hasattr(self.animal, attr):
return getattr(self.animal, attr)
else:
raise AttributeError(f"{type(self.animal)} does not have attribute {attr}")
def __getattribute__(self, attr):
if attr in ["speak", "run", "scratch"]:
return getattr(self.animal, attr)
else:
return super().__getattribute__(attr)
def main():
dog = Dog("Fido")
cat = Cat("Whiskers")
animals = [dog, cat]
for animal in animals:
animal_proxy = AnimalProxy(animal)
for attr in dir(animal_proxy):
if not attr.startswith("_"):
value = getattr(animal_proxy, attr)
if callable(value):
value()
else:
print(f"{attr}: {value}")
# 实现AnimalInterface接口的方法
class AnimalAdapter(AnimalInterface):
def __init__(self, animal):
self.animal = animal
def speak(self):
self.animal.speak()
def run(self):
self.animal.run()
def scratch(self):
if isinstance(self.animal, Cat):
self.animal.scratch()
else:
print(f"{type(self.animal)} cannot scratch")
cat_adapter = AnimalAdapter(cat)
cat_adapter.speak() # "Whiskers says meow"
cat_adapter.scratch() # "Whiskers is scratching"
if __name__ == "__main__":
main()
```
这段代码定义了一个`Animal`类和两个子类`Dog`和`Cat`。还定义了一个`AnimalInterface`接口,其中包含`speak`、`run`和`scratch`三个方法。然后定义了一个`AnimalProxy`类,它作为一个代理,用于获取动物对象的属性和方法。`AnimalProxy`实现了`__getattr__`和`__getattribute__`方法,用于获取动物对象中的属性和方法。
在`main`函数中,我们创建了一个`Dog`和一个`Cat`对象,并通过`AnimalProxy`来访问这些对象的属性和方法。然后,我们定义了一个适配器类`AnimalAdapter`,用于使`Cat`类实现`AnimalInterface`接口中的方法。在`main`函数中,我们创建了一个`AnimalAdapter`对象,将`Cat`对象传递给它,并调用`speak`和`scratch`方法。
这段代码演示了如何使用反射获取继承类的属性和方法,并调用这些属性和方法,以及实现接口的方法。
阅读全文
相关推荐



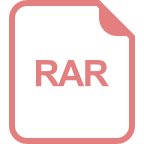
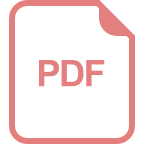
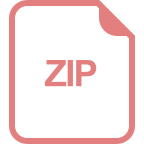
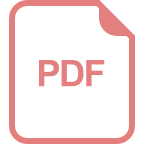
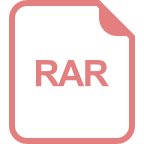
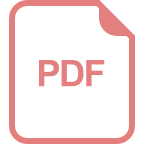
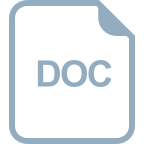
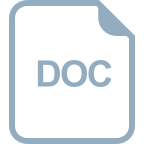
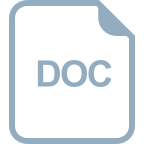
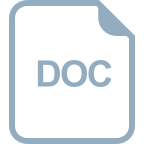
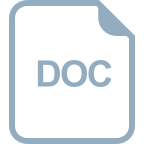
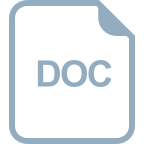
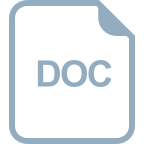