用python写一个判断某年某月有多少天
时间: 2023-05-16 19:06:42 浏览: 167
以下是用 Python 编写判断某年某月有多少天的代码:
```python
import calendar
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
days = calendar.monthrange(year, month)[1]
print(f"{year}年{month}月有{days}天")
```
这段代码使用了 Python 内置的 `calendar` 模块,其中 `monthrange(year, month)` 函数返回一个元组,第一个元素是该月第一天是星期几(0 表示星期一,6 表示星期日),第二个元素是该月的天数。我们只需要取出第二个元素即可。
注意:这段代码只能判断公历年份的天数,对于农历等其它历法需要另行处理。
相关问题
用python写一个判断某年某月天数
可以使用Python中的calendar模块来实现判断某年某月天数的功能。具体代码如下:
```python
import calendar
def is_leap_year(year):
"""
判断是否为闰年
"""
return calendar.isleap(year)
def get_days_in_month(year, month):
"""
获取某年某月的天数
"""
return calendar.monthrange(year, month)[1]
```
其中,`is_leap_year(year)`函数用于判断是否为闰年,如果是闰年则返回True,否则返回False。`get_days_in_month(year, month)`函数用于获取某年某月的天数,返回对应的天数值。使用方法示例如下:
```python
year = 2022
month = 2
if is_leap_year(year):
print(f"{year}年是闰年")
else:
print(f"{year}年不是闰年")
days = get_days_in_month(year, month)
print(f"{year}年{month}月共有{days}天")
```
输出结果为:
```
2022年不是闰年
2022年2月共有28天
```
希望能够帮到你!
利用python写一个输入某年,某月,某日判断这是这一年的多少天
好的,下面是实现代码:
```python
year = int(input("请输入年份:"))
month = int(input("请输入月份:"))
day = int(input("请输入日期:"))
# 计算是否为闰年
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
leap = True
else:
leap = False
# 计算天数
days = 0
for i in range(1, month):
if i in [1, 3, 5, 7, 8, 10, 12]:
days += 31
elif i == 2:
if leap:
days += 29
else:
days += 28
else:
days += 30
days += day
print("这是第{}天。".format(days))
```
运行代码,输入年月日后即可得到该日期是该年的第几天。
阅读全文
相关推荐
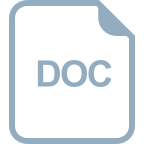
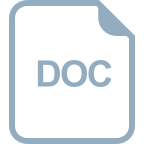
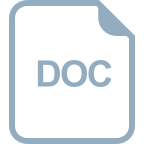













