采用自动轨迹绘制的方法,绘制一朵玫瑰花,写一段python代码
时间: 2024-01-31 16:01:50 浏览: 64
以下是使用Python和turtle库绘制一朵玫瑰花的代码:
```python
import turtle
# 设置画布大小和背景颜色
turtle.setup(600, 600)
turtle.bgcolor("black")
# 设置画笔颜色和线宽
turtle.color("red", "green")
turtle.pensize(2)
# 定义玫瑰花的绘制函数
def draw_rose():
for i in range(200):
turtle.speed(0)
turtle.right(1)
turtle.forward(1)
if i % 50 == 0:
turtle.right(90)
turtle.forward(20)
turtle.right(180)
turtle.forward(20)
turtle.right(90)
# 调用绘制函数,开始绘制
draw_rose()
# 隐藏画笔,保留绘制结果
turtle.hideturtle()
turtle.done()
```
运行以上代码,即可在画布上看到一朵玫瑰花的绘制结果。其中,绘制函数`draw_rose()`中的代码通过`turtle.right()`和`turtle.forward()`函数控制画笔的移动,实现了自动绘制的效果。
相关问题
你能帮我写一个python代码绘制一朵玫瑰花吗
当然可以,以下是一个简单的 Python 代码,可以绘制一朵玫瑰花:
```python
import turtle
# 设置画布大小和背景颜色
turtle.setup(800, 800)
turtle.bgcolor("black")
# 设置画笔颜色和线条宽度
turtle.color("red", "pink")
turtle.pensize(3)
# 绘制玫瑰花
turtle.begin_fill()
for i in range(200):
turtle.right(1)
turtle.forward(2)
if i % 50 == 0:
turtle.right(90)
turtle.forward(20)
turtle.right(180)
turtle.forward(20)
turtle.right(90)
turtle.end_fill()
# 隐藏画笔
turtle.hideturtle()
# 显示绘图窗口
turtle.done()
```
运行以上代码,即可在绘图窗口中看到一朵玫瑰花的绘制效果。
帮我写一段python代码,输出五个窗口,每个窗口都绘制一朵形态颜色各异的玫瑰花
在Python中,你可以使用matplotlib库来创建图形,而tkinter库用于创建窗口。这里是一个简单的例子,展示如何在一个程序中创建5个窗口,并在每个窗口中绘制不同颜色和形态的玫瑰花:
```python
import matplotlib.pyplot as plt
from tkinter import Tk, Canvas
# 创建一个函数,用于绘制单朵玫瑰花
def draw_roses(canvas, color, petal_count):
ax = canvas.figure.add_subplot(111)
ax.set_title(f"Rose with {petal_count} petals")
x = [i for i in range(petal_count)]
y = [1 - (abs(i - petal_count / 2) / (petal_count / 4)) for i in x]
roses = plt.plot(x, y, 'o', ms=10, color=color)
return roses
# 创建主函数,循环创建并显示5个窗口
def create_windows():
for i in range(5):
root = Tk()
width, height = 400, 400
root.geometry(f"{width}x{height}")
# 创建画布
canvas = Canvas(root, width=width, height=height)
canvas.pack()
# 绘制不同颜色的玫瑰花
colors = ['red', 'blue', 'green', 'yellow', 'purple']
petal_count = 12
roses = draw_roses(canvas, colors[i], petal_count)
root.mainloop() # 运行当前窗口事件循环
create_windows()
```
这个代码首先定义了一个`draw_roses`函数来绘制特定颜色和花瓣数目的玫瑰花。然后在`create_windows`函数中,创建了5个Tkinter窗口,每个窗口都有一个Canvas,我们在这个Canvas上调用`draw_roses`函数绘制不同颜色的玫瑰。
注意:运行此代码需要安装matplotlib和tkinter库,如果没有安装,可以分别通过pip install matplotlib和pip install tk来安装。
阅读全文
相关推荐
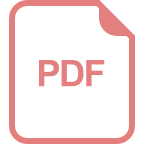
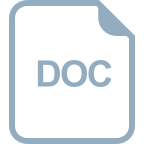
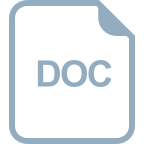











