上面的问题我希望用python实现,如果可以实现请给我一段dome
时间: 2024-04-04 14:31:55 浏览: 7
好的,下面是一个使用 Python 实现的示例代码,可以实现收集用户在手机上的操作行为,包括点击、滑动、输入等操作。
首先,你需要安装 Appium-Python-Client 和相关依赖库。可以通过 pip 命令来安装它们:
```
pip install Appium-Python-Client
pip install Appium-Python-Client[android]
```
然后,你需要启动一个 Appium 服务,并连接你的手机或模拟器。可以通过以下代码来启动 Appium 服务:
```python
from appium import webdriver
desired_caps = {
'platformName': 'Android',
'platformVersion': '10',
'deviceName': 'Android Emulator',
'appPackage': 'com.example.myapp',
'appActivity': 'MainActivity'
}
driver = webdriver.Remote('http://localhost:4723/wd/hub', desired_caps)
```
这里的 `desired_caps` 参数是一个字典,用于指定 Appium 的配置参数,例如指定要操作的设备、应用程序包名和启动 Activity 等。
接下来,你可以使用 Appium-Python-Client 提供的 API 来获取用户的操作行为。例如,你可以通过以下代码来获取用户在输入框中输入的文本:
```python
input_box = driver.find_element_by_id('com.example.myapp:id/input_box')
input_text = input_box.text
```
你还可以通过以下代码来模拟用户点击一个按钮:
```python
button = driver.find_element_by_id('com.example.myapp:id/button')
button.click()
```
如果你想要模拟用户滑动屏幕,可以使用 TouchAction 类,例如:
```python
from appium.webdriver.common.touch_action import TouchAction
action = TouchAction(driver)
action.press(x=100, y=500).move_to(x=100, y=100).release().perform()
```
这里的 `press` 方法表示按下屏幕,`move_to` 方法表示移动手指,`release` 方法表示松开手指。你可以根据需要调整这些参数。
最后,你需要关闭 Appium 服务:
```python
driver.quit()
```
完整的示例代码如下:
```python
from appium import webdriver
from appium.webdriver.common.touch_action import TouchAction
desired_caps = {
'platformName': 'Android',
'platformVersion': '10',
'deviceName': 'Android Emulator',
'appPackage': 'com.example.myapp',
'appActivity': 'MainActivity'
}
driver = webdriver.Remote('http://localhost:4723/wd/hub', desired_caps)
# 获取输入框中的文本
input_box = driver.find_element_by_id('com.example.myapp:id/input_box')
input_text = input_box.text
# 点击按钮
button = driver.find_element_by_id('com.example.myapp:id/button')
button.click()
# 模拟滑动屏幕
action = TouchAction(driver)
action.press(x=100, y=500).move_to(x=100, y=100).release().perform()
driver.quit()
```
这段代码演示了如何获取用户在输入框中输入的文本、点击按钮和模拟滑动屏幕的操作。你可以根据需要扩展它。需要注意的是,这里没有包括数据存储和隐私保护等功能,需要自行实现。
相关推荐
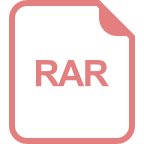














