用python写一个可以根据相机外参画出相机位姿示意图的程序
时间: 2023-04-09 15:04:45 浏览: 410
可以使用Python中的Matplotlib库来实现这个功能。以下是一个简单的示例代码:
```python
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
import numpy as np
# 相机内参
fx = 500
fy = 500
cx = 320
cy = 240
# 相机外参
R = np.array([[0.707, -0.707, 0], [0.707, 0.707, 0], [0, 0, 1]])
t = np.array([0, 0, 1])
# 生成相机坐标系的三个轴向量
Xc = np.array([1, 0, 0])
Yc = np.array([0, 1, 0])
Zc = np.array([0, 0, 1])
# 将相机坐标系的三个轴向量转换到世界坐标系中
Xw = R.dot(Xc)
Yw = R.dot(Yc)
Zw = R.dot(Zc)
# 绘制相机坐标系
fig = plt.figure()
ax = fig.add_subplot(111, projection='3d')
ax.quiver(t[0], t[1], t[2], Xw[0], Xw[1], Xw[2], color='r', length=0.2, normalize=True)
ax.quiver(t[0], t[1], t[2], Yw[0], Yw[1], Yw[2], color='g', length=0.2, normalize=True)
ax.quiver(t[0], t[1], t[2], Zw[0], Zw[1], Zw[2], color='b', length=0.2, normalize=True)
# 设置坐标轴范围
ax.set_xlim([-1, 1])
ax.set_ylim([-1, 1])
ax.set_zlim([0, 2])
# 设置坐标轴标签
ax.set_xlabel('X')
ax.set_ylabel('Y')
ax.set_zlabel('Z')
# 显示图像
plt.show()
```
这个程序可以根据相机内参和外参,绘制出相机坐标系的三个轴向量在世界坐标系中的示意图。其中,相机内参包括焦距和主点坐标,相机外参包括旋转矩阵和平移向量。绘制相机坐标系的三个轴向量时,需要将它们从相机坐标系转换到世界坐标系中。
阅读全文
相关推荐
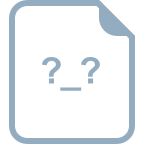
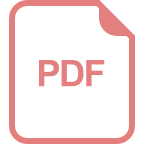
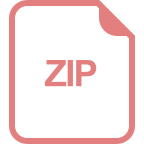



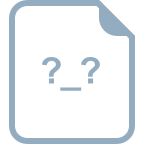
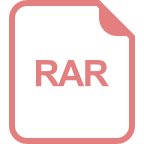
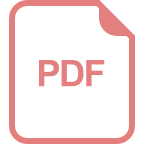
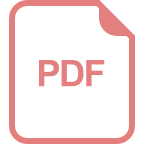
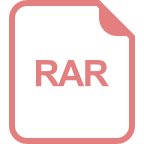
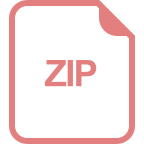
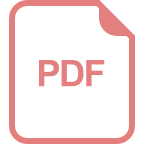