String sql = "select user_id from t_video where videoName=?"; pstm = connection.prepareStatement(sql); pstm.setString(1,"'"+videoName+"'");这段代码是否正确如果不正确怎么修改
时间: 2024-03-03 21:51:45 浏览: 69
这段代码不正确,应该修改为下面这样:
```
String sql = "select user_id from t_video where videoName=?";
pstm = connection.prepareStatement(sql);
pstm.setString(1, videoName);
```
在设置参数时,不需要将参数值用单引号包裹,而是直接将参数值传入 `setString` 方法中即可。
相关问题
public int updateStu(Student stu) throws Exception { Connection conn = null; PreparedStatement pstm = null; ResultSet rs = null; try { conn = dataSource.getConnection(); String sql = "update wn81.student set sname=? ,ssex=?,class=? where sno=?" ; pstm = conn.prepareStatement(sql); pstm.setString(1,stu.getSno()); pstm.setString(2,stu.getSname()); pstm.setString(3,stu.getSsex()); pstm.setString(4,stu.getCalss()); int i = pstm.executeUpdate(); Student s = null; return i ;这个方法有什么问题么
这个方法存在一些问题:
1. 在设置参数时,使用了错误的参数顺序。应该按照 SQL 语句中参数的顺序设置参数,而不是按照 `stu.getSno()`、`stu.getSname()`、`stu.getSsex()`、`stu.getCalss()` 的顺序设置参数。
2. 在 SQL 语句中,将 `sname` 和 `ssex` 的位置颠倒了。应该按照表结构中字段的顺序编写 SQL 语句。
3. 在执行更新操作后,没有对连接、语句和结果集进行关闭。应该使用 try-with-resources 或者 finally 块来关闭这些对象,以释放资源。
下面是修改后的代码:
```java
public int updateStu(Student stu) throws Exception {
try (Connection conn = dataSource.getConnection();
PreparedStatement pstm = conn.prepareStatement(
"UPDATE wn81.student SET sname=?, ssex=?, class=? WHERE sno=?")) {
pstm.setString(1, stu.getSname());
pstm.setString(2, stu.getSsex());
pstm.setString(3, stu.getCalss());
pstm.setString(4, stu.getSno());
int rowCount = pstm.executeUpdate();
return rowCount;
}
}
```
这个方法的参数是一个 `Student` 对象,但是在更新操作中没有使用 `stu` 的 `sno` 属性,因此可能导致更新错误。应该根据实际需求修改 SQL 语句,确保更新操作正确。
优化以下代码 :SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); String format = sdf.format(new Date()); format += " 00:00:01"; System.out.println(format); Connection connection = null; PreparedStatement pstm = null; ResultSet rs = null; OracleConnection1 oracleConnection1 = new OracleConnection1(); OracleConnection2 oracleConnection2 = new OracleConnection2(); connection = oracleConnection2.getConnection(); String SQLstr = "SELECT COUNT(1) FROM jy_xx_pkjf xx WHERE xx.jqrq >= to_date('"+format+"','yyyy-mm-dd hh24:mi:ss')"; int sum = 0; try { pstm = connection.prepareStatement(SQLstr); rs = pstm.executeQuery(SQLstr); if (rs.next()) { sum = rs.getInt(1); } } catch (SQLException throwables) { throwables.printStackTrace(); System.exit(0); } finally { oracleConnection2.ReleaseResource(); } String SQLstr2 = "INSERT INTO testi" + "nfo(frrq,gsdm,gsmc,zbs) values (sysdate,?,?,?)"; System.out.println(SQLstr2); connection = oracleConnection1.getConnection(); try { pstm = connection.prepareStatement(SQLstr2); pstm.setInt(1,123); pstm.setString(2,"神码"); pstm.setInt(3,sum); System.out.println(pstm); pstm.executeUpdate(); } catch (SQLException throwables) { throwables.printStackTrace(); } finally { oracleConnection2.ReleaseResource(); }
优化代码如下:
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
String formatDate = sdf.format(new Date());
String SQLstr = "SELECT COUNT(1) FROM jy_xx_pkjf xx WHERE xx.jqrq >= to_date('" + formatDate + "','yyyy-mm-dd hh24:mi:ss')";
System.out.println(SQLstr);
try (Connection connection = oracleConnection2.getConnection();
PreparedStatement pstm = connection.prepareStatement(SQLstr);
ResultSet rs = pstm.executeQuery(SQLstr)){
if (rs.next()) {
int sum = rs.getInt(1);
String SQLstr2 = "INSERT INTO testinfo(frrq,gsdm,gsmc,zbs) values (sysdate,?,?,?)";
System.out.println(SQLstr2);
try (PreparedStatement pstm2 = connection.prepareStatement(SQLstr2)) {
pstm2.setInt(1, 123);
pstm2.setString(2, "神码");
pstm2.setInt(3, sum);
System.out.println(pstm2);
pstm2.executeUpdate();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
} catch (SQLException throwables) {
throwables.printStackTrace();
} finally {
oracleConnection2.ReleaseResource();
}
优化的主要思路是:
1.将日期格式化的代码放在SQL语句字符串拼接时完成,不需要新建变量保存。
2.使用try-with-resources结构,简化代码结构,避免忘记关闭资源。
3.将数据库连接、执行SQL语句的代码放在同一个try块里面,减少出错的可能性。
4.将SQL语句2的执行代码放在SQL语句1的if块里面,当查询结果不为空时才进行插入操作,避免不必要的执行。
阅读全文
相关推荐
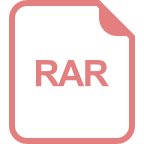
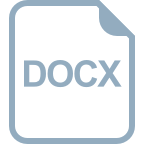



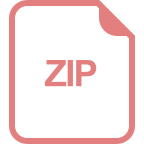
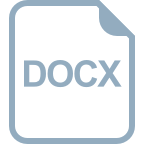
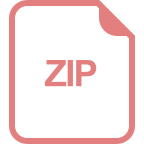
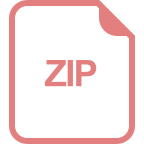
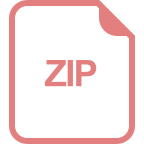
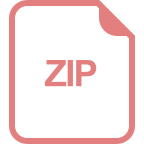
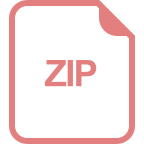
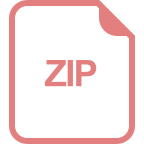
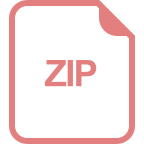