Unity资源加密方法有几种,代码怎么写
时间: 2024-11-07 22:29:38 浏览: 21
Unity引擎支持对资源文件进行加密,主要有两种常见的方法:
1. **Unity内置加密**:
使用Unity编辑器内置的AssetBundleEncryption工具可以对资源进行加密。这个工具允许你在项目设置中选择需要加密的AssetBundle,并输入密钥。加密后的资源在运行时需要相同的密钥才能解密加载。代码层面主要是通过`UnityEngine.Experimental.AssetManagement.AssetBundleManager` API来处理加密资产。
```csharp
using UnityEngine.Experimental.AssetManagement;
// ...
string encryptedAssetName = "EncryptedAsset";
string encryptionKey = "your_encryption_key"; // 这里替换为实际的加密密钥
// 加载加密AssetBundle
AssetBundle bundle = AssetBundle.LoadFromMemory(new byte[] { /* encrypted data from file or generated by tool */ }, encryptionKey);
bundle.LoadAssetAsync<GameObject>(encryptedAssetName).Then((asset) => {
if (asset != null)
Debug.Log("Loaded encrypted asset successfully.");
else
Debug.LogError("Failed to load encrypted asset.");
});
```
2. **自定义加密**:
如果你需要更高级别的安全性,可以编写自定义的加密算法,如AES(Advanced Encryption Standard)。但这通常涉及到更多的编码和数据处理工作。例如,你可以将资源内容转换成字节数组,然后使用加密算法进行处理,再存储为二进制流。
```csharp
using System.Security.Cryptography.AES;
// ...
byte[] encryptionKey = Encoding.UTF8.GetBytes("your_custom_key");
byte[] resourceData = File.ReadAllBytes("unencrypted_resource.abc");
// 创建AES加密器
Aes aes = Aes.Create();
aes.Key = encryptionKey;
ICryptoTransform encryptor = aes.CreateEncryptor(aes.Key, aes.IV);
// 加密数据
byte[] encryptedData = encryptor.TransformFinalBlock(resourceData, 0, resourceData.Length);
// 将加密数据保存到新文件
File.WriteAllBytes("encrypted_resource.encrypted", encryptedData);
```
阅读全文
相关推荐
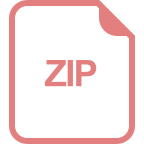
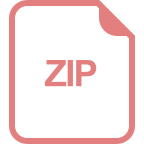
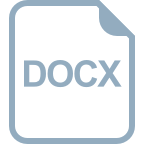
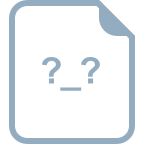
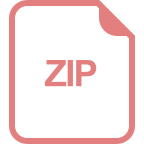
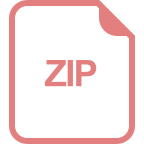
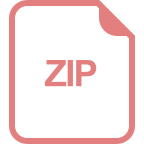
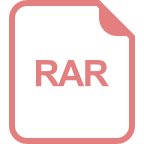
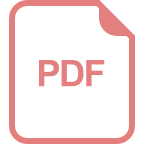
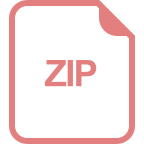
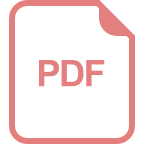
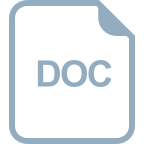
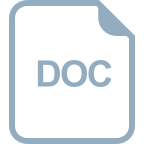
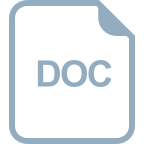
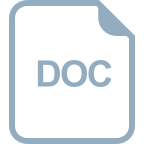
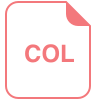
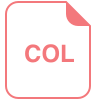
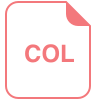
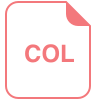