如何在C++中创建一个3x2的二维数组,填充随机数,然后计算并打印该矩阵的行列式?同时,找出并输出数组中最大数值的所在行号和列号?
时间: 2024-12-15 19:14:41 浏览: 15
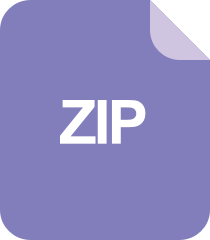
cpp代码-(二维数组)(1)随机数,赋值给二维数组(2)把主对角线副对角线的元素赋值给一维数组
在C++中,首先我们需要包含一些必要的库头文件,然后可以使用`std::vector`来动态创建二维数组,并填充随机数。接下来我们将演示如何计算行列式、找到最大值及其位置。
```cpp
#include <iostream>
#include <vector>
#include <ctime> // 用于生成随机数
#include <cstdlib> // 用于rand函数
// 用于计算3x3矩阵行列式的辅助函数
double determinant(std::vector<std::vector<int>>& matrix) {
if (matrix.size() == 2 && matrix[0].size() == 2) { // 3x2矩阵不需要行列式
return matrix[0][0] * matrix[1][1] - matrix[0][1] * matrix[1][0];
} else {
return 0; // 无法计算非3x3矩阵的行列式
}
}
int main() {
srand(time(0)); // 设置随机数种子,每次运行得到不同的随机数
int rows = 3;
int cols = 2;
std::vector<std::vector<int>> matrix(rows, std::vector<int>(cols));
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
matrix[i][j] = rand() % 100; // 生成0到99之间的随机整数
}
}
double det = determinant(matrix);
std::cout << "Matrix:\n";
for (const auto& row : matrix) {
for (int elem : row) {
std::cout << elem << " ";
}
std::cout << "\n";
}
std::cout << "Determinant: " << det << "\n";
int max_value = *std::max_element(matrix.begin(), matrix.end());
auto max_pos = std::max_element(matrix.begin(), matrix.end());
int max_row = max_pos.position() / cols;
int max_col = max_pos.position() % cols;
std::cout << "Max value (" << max_value << ") is at row: " << max_row+1 << ", column: " << max_col+1 << "\n";
return 0;
}
```
阅读全文
相关推荐
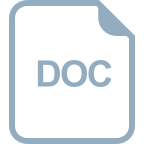
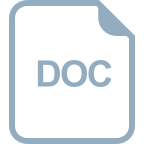
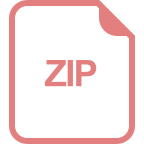
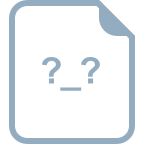
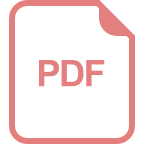
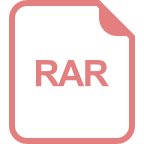
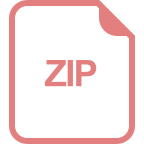
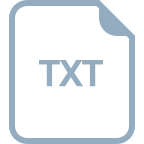
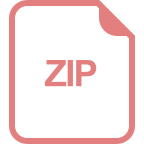
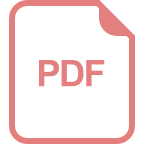
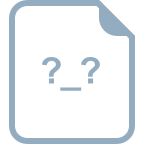
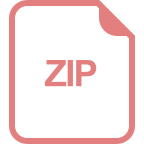
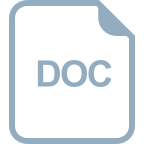
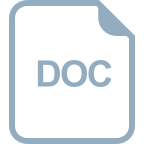
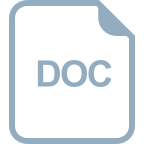