c++中有类似linkedhashmap的结构嘛
时间: 2024-09-10 07:13:41 浏览: 43
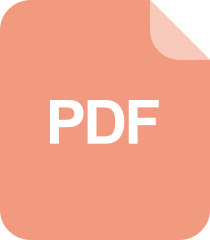
详解Java中LinkedHashMap
在C++标准库中,并没有直接提供一个与Java中的LinkedHashMap完全对应的容器。LinkedHashMap的特点是它不仅维护了元素的值,还维护了元素的插入顺序,这在C++的STL(标准模板库)中并没有现成的容器。
不过,我们可以使用C++中的其他数据结构组合来实现类似LinkedHashMap的功能。通常的做法是结合使用std::map(或std::unordered_map,取决于是否需要元素有序)和一个list(或forward_list)来模拟。这里的关键在于:
1. 使用map(或unordered_map)来存储键值对,这样可以保证键到值的映射以及高效的查找性能。
2. 使用list(或forward_list,取决于插入操作的频繁程度和是否需要双向迭代)来维护元素的插入顺序。
具体实现时,每次插入或访问map中的元素时,同时在list中插入(或移动到头部)该元素的迭代器,这样list中的元素顺序就对应了元素的插入或访问顺序。通过这种方式,我们可以模拟出LinkedHashMap的行为。
下面是一个简单的示例代码,使用std::map和std::list来模拟LinkedHashMap:
```cpp
#include <iostream>
#include <map>
#include <list>
#include <utility>
template <typename K, typename V>
class LinkedHashMap {
private:
std::list<std::pair<K, V>> orderList;
std::map<K, std::pair<V, typename std::list<std::pair<K, V>>::iterator>> dataMap;
public:
void put(const K& key, const V& value) {
auto it = dataMap.find(key);
if (it != dataMap.end()) {
orderList.erase(it->second.second);
it->second.first = value; // 更新值
} else {
it = dataMap.insert(std::make_pair(key, std::make_pair(value, orderList.end()))).first;
}
orderList.push_front(*it); // 插入到list头部
}
V get(const K& key) {
auto it = dataMap.find(key);
if (it != dataMap.end()) {
return it->second.first;
}
return V(); // 返回默认值
}
void remove(const K& key) {
auto it = dataMap.find(key);
if (it != dataMap.end()) {
orderList.erase(it->second.second);
dataMap.erase(it);
}
}
void display() {
for (const auto& pair : orderList) {
std::cout << pair.first << ": " << pair.second << std::endl;
}
}
};
int main() {
LinkedHashMap<int, std::string> map;
map.put(1, "one");
map.put(2, "two");
map.put(3, "three");
map.display(); // 按照插入顺序输出元素
return 0;
}
```
阅读全文
相关推荐
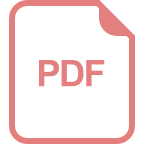
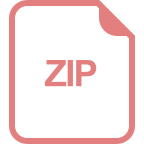















