.Linux循环获取系统时间
时间: 2024-08-21 07:01:07 浏览: 99
在 Linux 系统中,如果你想持续不断地获取当前的时间,你可以使用 Python 的 `time` 模块配合 `while` 循环来实现。下面是一个简单的例子:
```python
import time
# 获取当前时间的函数
def get_current_time():
return time.strftime("%Y-%m-%d %H:%M:%S", time.localtime())
while True:
print("当前时间为:", get_current_time())
# 可以设置一段时间(如秒、分钟)后再获取一次,这里假设每秒钟获取一次
time.sleep(1)
```
这段代码会不断地打印出当前的日期和时间,直到你停止运行它。如果你想要更精确地控制循环频率,可以修改 `time.sleep()` 中的参数。
相关问题
.Linux C循环获取系统时间
在 Linux 环境下,如果你想通过 C 语言编写程序来持续获取系统时间,你可以利用 `time()` 或者 `gettimeofday()` 函数。以下是两个示例:
1. 使用 `time()` 函数:
```c
#include <stdio.h>
#include <time.h>
int main() {
while (true) {
time_t current_time = time(NULL);
struct tm *timeinfo = localtime(¤t_time);
printf("当前时间: %d-%02d-%02d %02d:%02d:%02d\n",
timeinfo->tm_year + 1900, //转换为公元纪年
timeinfo->tm_mon + 1, //月份从0开始计数
timeinfo->tm_mday,
timeinfo->tm_hour,
timeinfo->tm_min,
timeinfo->tm_sec);
// 按照需要设置刷新频率,这里可以使用sleep函数暂停一段时间
sleep(1); // 每秒获取一次时间
}
return 0;
}
```
2. 使用 `gettimeofday()` 函数获取微秒级精度:
```c
#include <sys/time.h>
#include <unistd.h>
int main() {
struct timeval tv;
fd_set readfds;
while (true) {
gettimeofday(&tv, NULL);
printf("当前时间: %lu.%06lu 秒\n",
tv.tv_sec, tv.tv_usec); // tv_usec是微秒
FD_ZERO(&readfds);
FD_SET(STDIN_FILENO, &readfds);
select(STDIN_FILENO + 1, &readfds, NULL, NULL, &tv);
// 如果有输入事件,处理后继续循环;如果没有,则等待一秒后再检查
if (FD_ISSET(STDIN_FILENO, &readfds)) {
process_input();
} else {
usleep(1000 * 1000); // 每秒获取一次时间
}
}
return 0;
}
```
以上代码都会持续获取系统时间并显示出来,`select()` 可以让你在等待用户输入或其他事件的同时节省 CPU 资源。
写一个循环覆盖程序,检测盘小于3G开始删除最早的文件,文件目录结构时主目录/类型/子类型/日期/标识+时间.类型。在linux系统上,用c写
好的,以下是一个简单的循环覆盖程序的C代码,可以在Linux系统上运行。请注意,这个程序只是一个演示,实际应用中需要根据具体情况进行修改和完善。
```
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <dirent.h>
#include <sys/stat.h>
#include <unistd.h>
#define MAX_PATH 1024 // 文件路径最大长度
#define MAX_SIZE (3 * 1024 * 1024 * 1024) // 最大磁盘使用量,这里设为3GB
int main(int argc, char *argv[])
{
char root_path[MAX_PATH]; // 根目录路径
strcpy(root_path, argv[1]); // 获取根目录路径,命令行传入参数
DIR *root_dir = opendir(root_path); // 打开根目录
if (!root_dir) {
perror("Error opening root directory");
exit(EXIT_FAILURE);
}
struct dirent *entry;
struct stat file_stat;
char file_path[MAX_PATH]; // 文件路径
char oldest_file[MAX_PATH]; // 最早的文件路径
time_t oldest_time = time(NULL); // 最早的文件时间戳
int file_count = 0; // 文件数目
int dir_count = 0; // 目录数目
int delete_count = 0; // 删除的文件数目
off_t disk_usage = 0; // 磁盘使用量
// 遍历根目录下的所有文件和目录
while ((entry = readdir(root_dir)) != NULL) {
if (entry->d_name[0] == '.') // 跳过隐藏文件和目录
continue;
snprintf(file_path, MAX_PATH, "%s/%s", root_path, entry->d_name); // 拼接文件路径
if (stat(file_path, &file_stat) == -1) { // 获取文件信息
perror("Error getting file stat");
continue;
}
if (S_ISDIR(file_stat.st_mode)) { // 如果是目录,则递归遍历
dir_count++;
// 这里假设子目录的命名规则是“类型/子类型/日期/标识+时间.类型”
main(file_path); // 递归遍历子目录
} else if (S_ISREG(file_stat.st_mode)) { // 如果是普通文件
file_count++;
disk_usage += file_stat.st_size; // 累加磁盘使用量
if (difftime(file_stat.st_mtime, oldest_time) < 0) { // 如果比最早的文件还早
oldest_time = file_stat.st_mtime; // 更新最早的文件时间戳
strcpy(oldest_file, file_path); // 更新最早的文件路径
}
}
}
closedir(root_dir);
printf("Directory: %s\n", root_path);
printf("Directories: %d, Files: %d, Disk usage: %ld bytes\n", dir_count, file_count, disk_usage);
if (disk_usage > MAX_SIZE) { // 如果磁盘使用量超过最大值
printf("Disk usage exceeds maximum size, deleting oldest file: %s\n", oldest_file);
if (remove(oldest_file) == -1) { // 删除最早的文件
perror("Error deleting file");
exit(EXIT_FAILURE);
}
printf("File deleted\n");
delete_count++;
}
printf("Deleted %d files\n", delete_count);
return EXIT_SUCCESS;
}
```
这个程序的主要思路是递归遍历指定目录下的所有文件和子目录,统计磁盘使用量并记录最早的文件路径和时间戳。如果磁盘使用量超过最大值,就删除最早的文件。程序中假设子目录的命名规则是“类型/子类型/日期/标识+时间.类型”,如果实际情况不同,需要相应调整程序中的处理逻辑。
阅读全文
相关推荐
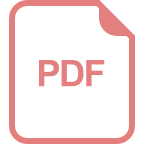
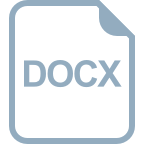
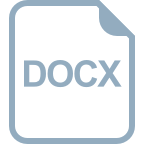
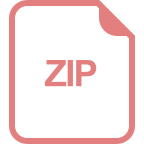
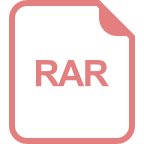
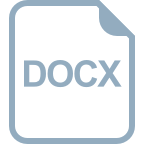
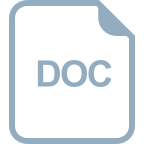
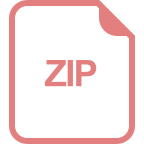
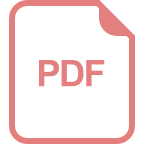
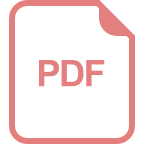
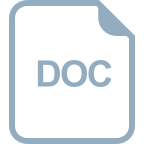
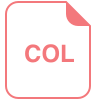
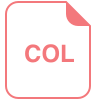
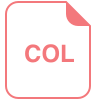
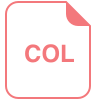
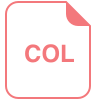
